Introduction
This page describes the Saferpay JSON application programming interface.
Our API is designed to have predictable, resource-oriented URLs and to use HTTP response codes to indicate API errors. We use built-in HTTP features, like HTTP authentication and HTTP verbs, which can be understood by off-the-shelf HTTP clients. JSON will be returned in all responses from the API, including errors.
Content Encoding
UTF-8
must be used for text encoding (there are restrictions on allowed characters for specific fields though).
Content-Type
and Accept
headers should be set to application/json
for server-to-server calls. Redirects use the standard browser types.
HTTP Headers:
Content-Type: application/json; charset=utf-8
Accept: application/json
Formats
The Saferpay JSON Api uses unified and standardized formats. The following abbreviations for format information are used in this page:
Name | Definition | Description |
---|---|---|
Id | A-Za-z0-9.:-_ | Alphanumeric with dot, colon, hyphen and underscore. |
Numeric | 0-9 | Numbers. |
Boolean | true or false | Boolean values. |
Date | ISO 8601 Date and Time | ISO 8601 format, e.g. 2007-12-24T18:21:25.123Z (UTC) or 2007-12-24T19:21:25.123+01:00 (CET). Max 3 digits in the fractional seconds part. |
Authentication
Saferpay supports the mechanism of basic authentication or a client certificate for authentication of a server (host) system.
Important: You must use either the Basic Authentication, OR the Client Certificate, but not both! Also make sure, that you do not send any faulty or old certificates, or authentication/accept headers. Otherwise our corporate Firewall will reject the call with a 403-Forbidden status! Furthermore, please note, that some environments do this by default. So even if you didn't implement it, the environment may do it as a default! It may be necessary to check your configuration.
HTTP basic authentication
This is the default authentication method. Technical users for the JSON API can be created by the merchant in the Saferpay Backoffice under Settings > JSON API basic authentication. The password will not be stored at SIX (only a securely salted hash thereof). There will be no password recovery besides creating a new user / password pair from your Backoffice account.
The password must meet some complexity requirements. We suggest using / generating dedicated passwords with a length of 16 alphanumeric characters (up to 32 characters).
HTTP Header:
Authorization: Basic [your base64 encoded "user_name:password"]
HTTPS Client Certificate Authentication
Alternatively, Saferpay also supports authentication via a client certificate.
Important: This feature is only available for Saferpay Business merchants!
A client certificate for the JSON-API can be ordered in the Saferpay Backoffice under Settings > JSON API client certificate.
If you have a corresponding license, you will find the HTTPS Client Certificate Authentication section under the form for HTTPS Basic Authentication.
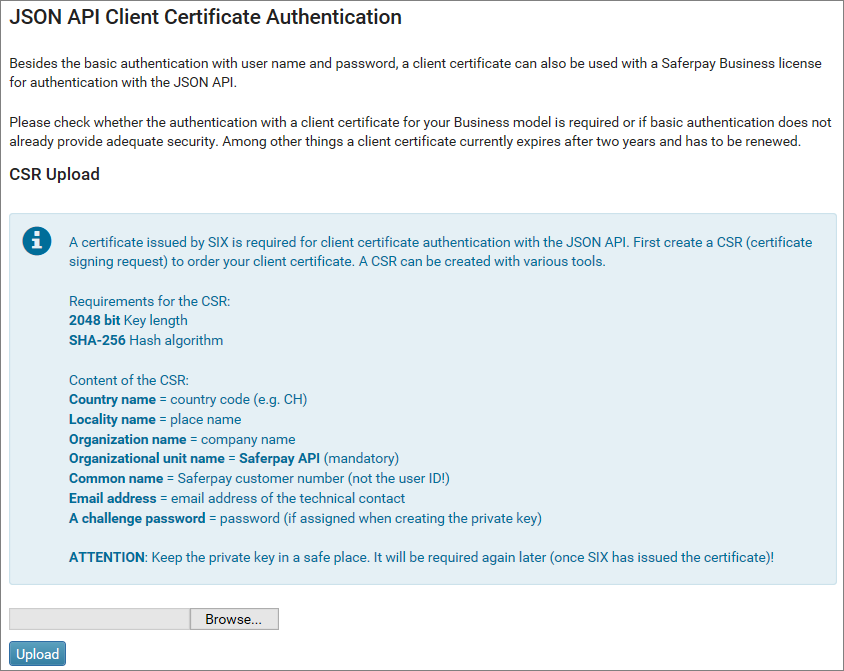
Generate the CSR as described on the page and import it using the upload button. The signed client certificate will then be downloaded through your browser.
Integration
Test Environment
For the integration phase, you should visit our test environment! There you can register your personal test account, which you then can use for testing, to try out different functions and for general evaluation.
You can find a list of test-cards and other payment means for testing over here!
Integration Guide
While these Documents are meant as a quick reference and technical specification of the Saferpay JSON-API, the Saferpay Integration Guide contains an in depth explanation about payment-flows, tips and tricks, as well as best practices for those, who want to integrate the JSON-API and its features for the first time. It will also help to understand certain characteristics about the different payment methods we offer, as well as the rules you must follow, when processing vital credit card data and more.
The sequential steps of the general integration process are described in our Step-by-step Integration-Manual.
Server-to-Server code Samples
The JSON API is a modern and lightweight interface, that can be used with all shop systems and all programming languages. Only a few steps are necessary to integrate your online shop with Saferpay. The proceeding is mostly as follows:
- Initialize via secure server-to-server call
- Integrate iframe to redirect your customer
- Authorize/ assert customer interaction via secure server-to-server call
In secure server-to-server calls you have to submit a JSON request containing you processing instructions to the defined URLs. The URL and the JSON structure varies depending on the action/resource you want to call. For further details check the description of resources below.
Server-to-server calls are a secure way to submit and gather data. Hence, a server-to-server call should always follow after the customer returns back to the shop, to gather information about the outcome of e.g. 3D Secure.
Important: Saferpay only supports TLS 1.2 and up, for secure connections. Please make sure, that your system is configured accordingly! More information in our TLS-FAQ.
Important: The redirect towards the redirectUrl via http-POST IS NOT supported. You should always use http-GET, unless specifically stated otherwise!
Caution: Please DO NOT use client side scripting languages like JavaScript to execute the server-to-server (JSON) calls directly. Clients could manipulate the code, or even try to extract the credentials, to execute refunds or other requests with malicious intentions. Always execute the requests and keep your credentials on your server, maybe by using AJAX.
Caution: DO NOT implement a polling-process, to poll for the transaction-data. Respond with the necessary request, at the correct time (e.g. doing the assert only, if the SuccessUrl, or NotifyUrl are called). Saferpay reserves the right to otherwise deactivate, or block your account!
Server-to-server communication:
private object SubmitRequest(string sfpUrl, object request, string sfpLogin, string sfpPassword) { // don't keep your connection alive, it's a simple request/response server call // for details on NoKeepAliveWebClient, see https://github.com/saferpay/jsonapi/blob/master/snippets/NoKeepAliveWebClient.cs using (var client = new NoKeepAliveWebClient()) { string authInfo = $"{sfpLogin}:{sfpPassword}"; client.Headers[HttpRequestHeader.Authorization] = "Basic " + Convert.ToBase64String(Encoding.UTF8.GetBytes(authInfo)); client.Headers[HttpRequestHeader.Accept] = "application/json"; client.Headers[HttpRequestHeader.ContentType] = "application/json; charset=utf-8"; client.Encoding = Encoding.UTF8; try { var responseData = client.UploadString(sfpUrl, JsonConvert.SerializeObject(request)); return JsonConvert.DeserializeObject(responseData); } catch (WebException we) { if (we.Response is HttpWebResponse response) { Trace.WriteLine($"Web exception occured: {response.StatusCode} {response.StatusDescription}"); if (response.ContentLength > 0) { using (var rs = we.Response.GetResponseStream()) using (var sr = new StreamReader(rs)) { Trace.WriteLine($"{sr.ReadToEnd()}"); } } } else { Trace.WriteLine($"Web exception occured: {we.Message} ({we.Status}"); } throw; } } }
public static JsonObject sendRequest(URL sfpUrl, JsonObject request, String sfpLogin, String sfpPassword) throws IOException { //encode credentials String credential = sfpLogin + ":" + sfpPassword; String encodedCredentials = DatatypeConverter.printBase64Binary(credential.getBytes());//create connection HttpURLConnection connection = (HttpURLConnection) sfpUrl.openConnection(); connection.setRequestProperty("connection", "close"); connection.setRequestProperty("Content-Type", "application/json; charset=utf-8"); connection.setRequestProperty("Accept", "application/json"); connection.setRequestProperty("Authorization", "Basic " + encodedCredentials); connection.setRequestMethod("POST"); connection.setDoOutput(true); connection.setUseCaches(false); //write JSON to output stream JsonWriter writer = Json.createWriter(connection.getOutputStream()); writer.writeObject(request); writer.close(); //send request int responseCode = connection.getResponseCode(); //get correct input stream InputStream readerStream = responseCode == 200 ? connection.getInputStream() : connection.getErrorStream(); JsonObject response = Json.createReader(readerStream).readObject(); return response;
}
//This is an EXAMPLE of the payload-Array. $payload = array( 'RequestHeader' => array( 'SpecVersion' => "[current Spec-Version]", 'CustomerId' => "[YOUR CUSTOMERID]", 'RequestId' => "aScdFewDSRFrfas2wsad3", 'RetryIndicator' => 0, 'ClientInfo' => array( 'ShopInfo' => "My Shop", 'OsInfo' => "Windows Server 2013" ) ), 'TerminalId' => "[YOUR TERMINALID]", 'PaymentMethods' => array("DIRECTDEBIT","VISA"), 'Payment' => array( 'Amount' => array( 'Value' => "21065", 'CurrencyCode' => "EUR" ), 'OrderId' => "123test", 'PayerNote' => "A Note", 'Description' => "Test_Order_123test" ), 'Payer' => array( 'IpAddress' => "192.168.178.1", 'LanguageCode' => "en" ), 'ReturnUrl' => array( 'Url' => "[your shop payment url]" ), 'Notification' => array( 'PayerEmail' => "payee@mail.com", 'MerchantEmails' => array("merchant@mail.com"), 'SuccessNotifyUrl' => "[your success notify url]", 'FailNotifyUrl' => "[your fail notify url]" ), 'DeliveryAddressForm' => array( 'Display' => true, 'MandatoryFields' => array("CITY","COMPANY","COUNTRY","EMAIL","FIRSTNAME","LASTNAME","PHONE","SALUTATION","STATE","STREET","ZIP") ) ); //$username and $password for the http-Basic Authentication //$url is the SaferpayURL eg. https://www.saferpay.com/api/Payment/v1/PaymentPage/Initialize //$payload is a multidimensional array, that assembles the JSON structure. Example see above function do_curl($username,$password,$url, $payload){ //Set Options for CURL $curl = curl_init($url); curl_setopt($curl, CURLOPT_HEADER, false); //Return Response to Application curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); //Set Content-Headers to JSON curl_setopt($curl, CURLOPT_HTTPHEADER,array("Content-type: application/json","Accept: application/json; charset=utf-8")); //Execute call via http-POST curl_setopt($curl, CURLOPT_POST, true); //Set POST-Body //convert DATA-Array into a JSON-Object curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($payload)); //WARNING!!!!! //This option should NOT be "false", otherwise the connection is not secured //You can turn it of if you're working on the test-system with no vital data //PLEASE NOTE: //Under Windows (using WAMP or XAMP) it is necessary to manually download and save the necessary SSL-Root certificates! //To do so, please visit: http://curl.haxx.se/docs/caextract.html and Download the .pem-file //Then save it to a folder where PHP has write privileges (e.g. the WAMP/XAMP-Folder itself) //and then put the following line into your php.ini: //curl.cainfo=c:\path\to\file\cacert.pem curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, true); curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, 2); //HTTP-Basic Authentication for the Saferpay JSON-API. //This will set the authentication header and encode the password & username in Base64 for you curl_setopt($curl, CURLOPT_USERPWD, $username . ":" . $password); //CURL-Execute & catch response $jsonResponse = curl_exec($curl); //Get HTTP-Status //Abort if Status != 200 $status = curl_getinfo($curl, CURLINFO_HTTP_CODE); if ($status != 200) { //IF ERROR //Get http-Body (if aplicable) from CURL-response $body = json_decode(curl_multi_getcontent($curl), true); //Build array, containing the body (Response data, like Error-messages etc.) and the http-status-code $response = array( "status" => $status . " <|> " . curl_error($curl), "body" => $body ); } else { //IF OK //Convert response into an Array $body = json_decode($jsonResponse, true); //Build array, containing the body (Response-data) and the http-status-code $response = array( "status" => $status, "body" => $body ); } //IMPORTANT!!! //Close connection! curl_close($curl); //$response, again, is a multi-dimensional Array, containing the status-code ($response["status"]) and the API-response (if available) itself ($response["body"]) return $response; }
If you include the redirect pages into your web-page using an iframe, you can react on size changes of the iframe content by listening to a message event containing the new sizing information.
Please note: Depending on the bank, issuer, or payment provider, the page can try to break out of the iframe or lack telling you the actual size of the content.
Handle JavaScript events from Saferpay (for JQuery 1.9 and higher):
$(window).bind('message', function (e) {
switch (e.originalEvent.data.message) {
case 'css':
$('#iframe').css('height', e.originalEvent.data.height + "px");
break;
}
});
Granting IP-permission
As an additional security feature, you can also grant permissions to specific IPs. This way you can control the API access even further in connection with the authentication credentials. To do so, you need to log into the Saferpay Backoffice, for either production or test, then go under Settings > IP permissions and enter the IP, or IP range of your network/server!
Note: This feature is entirely optional and only supports IPv4 addresses only!
Building the correct API URL
Every request is differentiated by its own unique request URL. This way Saferpay knows which API-function you want to access. Combined with the base URL for either the production- or test-environment, you will get the complete API-URL. Here is an example of how to build this URL correctly:
Base URL production system:
https://www.saferpay.com/api
Base URL test system:
https://test.saferpay.com/api
Take the correct request URL and add it to the base URL. You can find it on the right side of the request-specification.
For instance, if you want to call PaymentPage Initialize, the request URL is:
/Payment/v1/PaymentPage/Initialize
Add this to the base URL and you will get the full API URL:
Full API URL production system:
https://www.saferpay.com/api/Payment/v1/PaymentPage/Initialize
Full API URL test system:
https://test.saferpay.com/api/Payment/v1/PaymentPage/Initialize
Error Handling
Successfully completed requests are confirmed with an http status code of 200
and
contain the appropriate response message in the body.
If the request could not be completed successfully, this is indicated by a status code
of 400
or higher and – if possible (some errors are generated by the web server itself,
or the web application firewall and are thus outside of our control) – an error message
stating the reason of the failure is included in the body of the response. The presence
of an error message as specified in this document can be derived from the content type:
if it’s application/json
, then there is an error message present.
HTTP status codes:
200 | OK (No error) |
400 | Validation error |
401 | Authentication of the request failed |
402 | Requested action failed |
403 | Access denied |
406 | Not acceptable (wrong accept header) |
415 | Unsupported media type (wrong content-type header) |
500 | Internal error |
Info: The API timeout for requests should be 100 seconds! After that, a connection should be closed. Also, no further actions should be taken, until the request is answered or runs into a timeout, to prevent unwanted behavior.
Error Message
Arguments | |
---|---|
Behavior mandatory, string |
What can be done to resolve the error?
Possible values: DO_NOT_RETRY, OTHER_MEANS, RETRY, RETRY_LATER. |
ErrorDetail array of strings |
More details, if available. Contents may change at any time, so don’t parse it.
|
ErrorMessage mandatory, string |
Description of the error. The contents of this element might change without notice, so do not parse it.
|
ErrorName mandatory, string |
Name / id of the error. These names will not change, so you may parse these and attach your logic to the ErrorName.
|
OrderId string |
OrderId of the failed transaction. This is only returned in the PaymentPage Assert Response and the Transaction Authorize Response.
|
PayerMessage string |
A text message provided by the card issuer detailing the reason for a declined authorization. It is safe to display it to the payer.
|
ProcessorMessage string |
Message returned by acquirer or processor
|
ProcessorName string |
Name of acquirer (if declined by acquirer) or processor
|
ProcessorResult string |
Result code returned by acquirer or processor
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Risk object |
Contains additional risk related information for the transaction that is blocked by risk.
|
TransactionId string |
Id of the failed transaction, if available
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Behavior": "DO_NOT_RETRY", "ErrorName": "VALIDATION_FAILED", "ErrorMessage": "Request validation failed", "ErrorDetail": [ "PaymentMeans.BankAccount.IBAN: The field IBAN is invalid." ], "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "OrderId": "c52ad18472354511ab2c33b59e796901" }
List of behaviors:
DO_NOT_RETRY | Do not try again to avoid potential fees related to authorization reattempts that do not respect card schemes instructions. The card issuer will never approve this authorization request. |
RETRY | Request is valid and understood, but can't be processed at this time. This request can be retried. |
RETRY_LATER | This request can be retried later after a certain state/error condition has been changed. For example, insufficient funds (up to 10 attempts in 30 days) |
OTHER_MEANS | Special case of retry. Please provide another means of payment. |
List of error names (these names will not change, so you may parse these and attach your logic to the ErrorName):
ACTION_NOT_SUPPORTED | The requested action is not supported in the given context or the action can't be executed with the request data. |
ALIAS_INVALID |
The alias is not known or already used (in case of registration). Solution: Use another alias for registration |
AMOUNT_INVALID | The amount does not adhere to the restrictions for this action. E.g. it might be exceeding the allowed capture amount. |
AUTHENTICATION_FAILED |
Wrong password, wrong client certificate, invalid token, wrong HMAC. Solution: Use proper credentials, fix HMAC calculation, use valid token |
BLOCKED_BY_RISK_MANAGEMENT |
Action blocked by risk management Solution: Unblock in Saferpay Risk Management (Backoffice) |
CARD_CHECK_FAILED |
Invalid card number or cvc (this is only returned for the SIX-internal chard check feature for Alias/InsertDirect). Solution: Let the card holder correct the entered data |
CARD_CVC_INVALID |
Wrong cvc entered Solution: Retry with correct cvc |
CARD_CVC_REQUIRED |
Cvc not entered but required Solution: Retry with cvc entered |
COMMUNICATION_FAILED |
The communication to the processor failed. Solution: Try again or use another means of payment |
COMMUNICATION_TIMEOUT |
Saferpay did not receive a response from the external system in time. It’s possible that an authorization was created, but Saferpay is not able to know this. Solution: Check with the acquirer if there is an authorization which needs to be canceled. |
CONDITION_NOT_SATISFIED | The condition which was defined in the request could not be satisfied. |
CURRENCY_INVALID | Currency does not match referenced transaction currency. |
GENERAL_DECLINED | Transaction declined by unknown reason |
INTERNAL_ERROR |
Internal error in Saferpay Solution: Try again |
NO_CONTRACT |
No contract available for the brand / currency combination. Solution: Use another card or change the currency to match an existing contract or have the currency activated for the contract. |
NO_CREDITS_AVAILABLE |
No more credits available for this account. Solution: Buy more transaction credits |
PAYER_AUTHENTICATION_REQUIRED | Payer authentication required to proceed (soft decline). |
PAYMENTMEANS_INVALID | Invalid means of payment (e.g. invalid card) |
PAYMENTMEANS_NOT_SUPPORTED | Unsupported means of payment (e.g. non SEPA IBAN) |
PERMISSION_DENIED | No permission (e.g. terminal does not belong to the customer) |
3DS_AUTHENTICATION_FAILED |
3D-secure authentication failed – the transaction must be aborted. Solution: Use another card or means of payment |
TOKEN_EXPIRED |
The token is expired. Solution: Create a new token. |
TOKEN_INVALID | The token either does not exist for this customer or was already used |
TRANSACTION_ABORTED | The transaction was aborted by the payer. |
TRANSACTION_ALREADY_CAPTURED | Transaction already captured. |
TRANSACTION_DECLINED |
Declined by the processor. Solution: Use another card or check details. |
TRANSACTION_IN_WRONG_STATE | |
TRANSACTION_NOT_FOUND | |
TRANSACTION_NOT_STARTED |
The transaction was not started by the payer. Therefore, no final result for the transaction is available. Solution: Try again later. |
UNEXPECTED_ERROR_BY_ACQUIRER |
The acquirer returned an unexpected error code. Solution: Try again |
UPDATE_CARD_INFORMATION |
Card details need to be updated in order to have the possibility of a successful payment Solution: Update card data |
VALIDATION_FAILED |
Validation failed. Solution: Fix request |
Payment Page
The Payment Page Interface provides a simple and easy integration of Saferpay into your web shop, mobile app or other applications without the need to implement a user interface for card data entry. Lincenses including the payment page can be found here. It allows the processing of all payment methods that are available with Saferpay. Once integrated, more payment methods can be activated at any time and without major adjustments.
Payment Process with the Payment Page
This chapter will give you a simple overview about the transaction flow, when using the Payment Page
Important Note: If you have trouble understanding the transaction flow with the Payment Page in its detail, you may want to read our Saferpay Integration guide, which offers an in depth explanation on how to integrate the Payment Page, optional features, best practices and more.
Transaction-flow
- Payment Page Initialize
- Initializes the Payment and generates the RedirectUrl for the Payment Page.
- Redirect to the RedirectUrl
- Return to ReturnUrl. The ReturnUrl is defined in step 1!
- Payment Page Assert
- Gathers all the information about the payment, like LiabilityShift through 3D Secure and more, using the Token, gathered in step 1!
- Depending on the outcome of step 4 you may
- Transaction is finished!
PaymentPage Initialize
POST
This method can be used to start a transaction with the Payment Page which may involve either DCC and / or 3d-secure
Request URL:
POST: /Payment/v1/PaymentPage/Initialize
Request
Arguments | |
---|---|
Authentication object |
Strong Customer Authentication (exemptions, ...)
|
BillingAddressForm object |
Use this container if you need to get a billing address from the payer during the payment process.
Saferpay can show an address form and depending on the means of payment, it is also possible to get the address from the means of payment (e.g. PayPal or any kind of wallet) if available. Check the options in the container for different behaviors. In case you also provide payer addresses, these are used as default values to prefill the address form (if displayed) and are overwritten by the final address entered by the payer or provided by the payment method. |
CardForm object |
Options for card data entry form (if applicable)
|
Condition string |
Optional Condition for Authorization (only 3DSv2), to control, whether or not, transactions without LiabilityShift should be accepted. Important Note: This only filters out transactions, where the condition is conclusive before the authorization itself. It is possible, that LiabilityShift is rejected after the authorization. Please always check the Liability container, within the authorization-response, to be 100% sure, if LiabilityShift applies, or not!
Possible values: NONE, THREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED.Default: NONE (empty) |
ConfigSet string |
This parameter let you define your payment page config (PPConfig) by name. If this parameters is not set, your default PPConfig will be applied if available.
Id[1..20]When the PPConfig can't be found (e.g. wrong name), the Saferpay basic style will be applied to the payment page. Example: name of your payment page config (case-insensitive)
|
DeliveryAddressForm object |
Use this container if you need to get a delivery address from the payer during the payment process.
Saferpay can show an address form and depending on the means of payment, it is also possible to get the address from the means of payment (e.g. PayPal or any kind of wallet) if available. Check the options in the container for different behaviors. In case you also provide payer addresses, these are used as default values to prefill the address form (if displayed) and are overwritten by the final address entered by the payer or provided by the payment method. |
Notification object |
Notification options
|
Order object |
Optional order information. Only used for payment method Klarna (mandatory) and for Fraud Intelligence (optional).
|
Payer object |
Information about the payer
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
PaymentMethods array of strings |
Used to restrict the means of payment which are available to the payer for this transaction. If only one payment method id is set, the payment selection step will be skipped.
Possible values: ACCOUNTTOACCOUNT, ALIPAY, AMEX, BANCONTACT, BONUS, DINERS, CARD, DIRECTDEBIT, EPRZELEWY, EPS, GIROPAY, IDEAL, INVOICE, JCB, KLARNA, MAESTRO, MASTERCARD, MYONE, PAYCONIQ, PAYDIREKT, PAYPAL, POSTFINANCEPAY, SOFORT, TWINT, UNIONPAY, VISA, WECHATPAY, WLCRYPTOPAYMENTS.Example: ["VISA", "MASTERCARD"]
|
PaymentMethodsOptions object |
Optional. May be used to set specific options for some payment methods.
|
RegisterAlias object |
If the given means of payment should be stored in Saferpay Secure Card Data storage (if applicable)
|
RequestHeader mandatory, object |
General information about the request.
|
ReturnUrl mandatory, object |
URL which is used to redirect the payer back to the shop
This Url is used by Saferpay to redirect the shopper back to the merchant shop. You may add query string parameters to identify your session, but please be aware that the shopper could modify these parameters inside the browser! The whole url including query string parameters should be as short as possible to prevent issues with specific browsers and must not exceed 2000 characters. Note: you should not add sensitive data to the query string, as its contents is plainly visible inside the browser and will be logged by our web servers. |
RiskFactors object |
Optional risk factors
|
TerminalId mandatory, string |
Saferpay terminal id
Numeric[8..8]Example: 12345678
|
Wallets array of strings |
Used to control if wallets should be enabled on the payment selection page.
Possible values: APPLEPAY, GOOGLEPAY.Example: ["APPLEPAY"]
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "OrderId": "Id of the order", "Description": "Description of payment" }, "ReturnUrl": { "Url": "[your shop payment url]" } }
Response
Arguments | |
---|---|
Expiration mandatory, date |
Expiration date / time of the RedirectUrl in ISO 8601 format in UTC. After this time, the RedirectUrl can't be called anymore.
Example: 2011-07-14T19:43:37+01:00
|
RedirectUrl mandatory, string |
RedirectUrl for the payment page transaction. Simply add this to a "Pay Now"-button or do an automatic redirect.
Example: https://www.saferpay.com/vt2/api/PaymentPage/1234/12341234/234uhfh78234hlasdfh8234e1234
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Token mandatory, string |
Token for later referencing
Example: 234uhfh78234hlasdfh8234e1234
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "Id of the request" }, "Token": "234uhfh78234hlasdfh8234e1234", "Expiration": "2015-01-30T12:45:22.258+01:00", "RedirectUrl": "https://www.saferpay.com/vt2/api/..." }
PaymentPage Assert
POST
Call this function to safely check the status of the transaction from your server.
Important:
- Depending on the payment provider, the resulting transaction may either be an authorization or may already be captured (meaning the financial flow was already triggered). This will be visible in the status of the transaction container returned in the response.
- This function can be called up to 24 hours after the transaction was initialized. For pending transaction the token expiration is increased to 120 hours.
- If the transaction failed (the payer was redirected to the Fail url or he manipulated the return url), an error response with an http status code 400 or higher containing an error message will be returned providing some information on the transaction failure.
Request URL:
POST: /Payment/v1/PaymentPage/Assert
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
Token mandatory, string |
Token returned by initial call.
Id[1..50]Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "Token": "234uhfh78234hlasdfh8234e" }
Response
Arguments | |
---|---|
Dcc object |
Dcc information, if applicable
|
FraudPrevention object |
Contains details of a performed fraud prevention check
|
Liability object |
LiabilityShift information, replaces ThreeDs Info from api version 1.8
|
MastercardIssuerInstallments object |
Mastercard card issuer installment payment options, if applicable
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
RegistrationResult object |
Information about the SCD registration outcome
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test Card", "AcquirerReference": "000000", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } }, "Liability": { "LiabilityShift": true, "LiableEntity": "THREEDS", "ThreeDs": { "Authenticated": true, "Xid": "ARkvCgk5Y1t/BDFFXkUPGX9DUgs=" } } }
Transaction
Payment Process with the Transaction Interface
This chapter will give you a simple overview about the general transaction flow, when using the Transaction Interface.
Important Note: The Transaction Interface offers all sorts of options to perform transactions. This flow only describes the general flow. Furthermore, you may want to read our Saferpay Integration guide, which offers an in depth explanation on how to integrate the Transaction Interface, optional features, best practices and more.
Transaction-flow
- Transaction Initialize
- Initializes the Payment and generates the RedirectUrl for the iFrame Integration.
- Open the RedirectUrl inside an HTML-iFrame, to show the hosted card entry form!
- Return to ReturnUrl. The ReturnUrl is defined in step 1!
- Transaction Authorize
- Authorizes the card, which has been gathered in step 2. Up until now, no transaction has been made!
- Depending on the outcome of step 4 you may
- Transaction is finished!
Transaction Initialize Available depending on license
POST
This method may be used to start a transaction which may involve either DCC and / or 3d-secure.
Warning: Only PCI certified merchants may submit the card-data directly, or use their own HTML form! Click here for more information!
Request URL:
POST: /Payment/v1/Transaction/Initialize
Request
Arguments | |
---|---|
Authentication object |
Strong Customer Authentication (exemptions, ...)
|
CardForm object |
Options for card data entry form (if applicable)
|
ConfigSet string |
This parameter let you define your payment page config (PPConfig) by name. If this parameters is not set, your default PPConfig will be applied if available.
Id[1..20]When the PPConfig can't be found (e.g. wrong name), the Saferpay basic style will be applied to the payment page. Example: name of your payment page config (case-insensitive)
|
Notification object |
Notification options
|
Order object |
Optional order information. Only used for payment method Klarna (mandatory) and for Fraud Intelligence (optional).
|
Payer object |
Information on the payer (IP-address)
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
PaymentMeans object |
Means of payment (either card data or a reference to a previously stored card).
Important: Only fully PCI certified merchants may directly use the card data. If your system is not explicitly certified to handle card data directly, then use the Saferpay Secure Card Data-Storage instead. If the customer enters a new card, you may want to use the Saferpay Hosted Register Form to capture the card data through Saferpay. |
PaymentMethods array of strings |
Used to restrict the means of payment which are available to the payer
Possible values: AMEX, BANCONTACT, BONUS, DINERS, DIRECTDEBIT, JCB, MAESTRO, MASTERCARD, MYONE, VISA. Additional values may be accepted but are ignored.Example: ["VISA", "MASTERCARD"]
|
RedirectNotifyUrls object |
If a redirect of the payer is required, these URLs will be used by Saferpay to notify you when the payer has completed the required steps and the transaction is ready to be authorized or when the operation has failed or has been aborted by the payer.
If no redirect of the payer is required, then these URLs will not be called (see RedirectRequired attribute of the Transaction Initialize response). Supported schemes are http and https. You also have to make sure to support the GET-method. The whole url including query string parameters must not exceed 2000 characters. Note: you should not add sensitive data to the query string, as its contents are logged by our web servers. |
RequestHeader mandatory, object |
General information about the request.
|
ReturnUrl mandatory, object |
URL which is used to redirect the payer back to the shop if the transaction requires some kind of browser redirection (3d-secure, dcc)
This Url is used by Saferpay to redirect the shopper back to the merchant shop. You may add query string parameters to identify your session, but please be aware that the shopper could modify these parameters inside the browser! The whole url including query string parameters should be as short as possible to prevent issues with specific browsers and must not exceed 2000 characters. Note: you should not add sensitive data to the query string, as its contents is plainly visible inside the browser and will be logged by our web servers. |
RiskFactors object |
Optional risk factors
|
Styling object |
Styling options
|
TerminalId mandatory, string |
Saferpay terminal to use for this authorization
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" } }, "Payer": { "LanguageCode": "en" }, "ReturnUrl": { "Url": "[your shop payment url]" }, "Styling": { "CssUrl": "[your shop css url]" } }
Response
Arguments | |
---|---|
Expiration mandatory, date |
Expiration date / time of the generated token in ISO 8601 format in UTC.
Example: After this time is exceeded, the token will not be accepted for any further actions except Asserts. 2015-01-30T13:45:22.258+02:00
|
LiabilityShift boolean |
Indicates if liability shift to issuer is possible or not. Not present if PaymentMeans container was not present in InitializeTransaction request. True, if liability shift to issuer is possible, false if not.
|
Redirect object |
Mandatory if RedirectRequired is true. Contains the URL for the redirect to use for example the Saferpay hosted register form.
|
RedirectRequired mandatory, boolean |
True if a redirect must be performed to continue, false otherwise
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Token mandatory, string |
Id for referencing later
Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Token": "234uhfh78234hlasdfh8234e", "Expiration": "2015-01-30T12:45:22.258+01:00", "LiabilityShift": true, "RedirectRequired": true, "Redirect": { "RedirectUrl": "https://www.saferpay.com/vt2/Api/...", "PaymentMeansRequired": true } }
Transaction Authorize Available depending on license
POST
This function may be called to authorize a transaction which was started by a call to Transaction/Initialize.
Request URL:
POST: /Payment/v1/Transaction/Authorize
Request
Arguments | |
---|---|
Condition string |
THREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED: the authorization will be executed if the previous 3d-secure process indicates that the liability shift to the issuer is possible
Possible values: NONE, THREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED.(liability shift may still be declined with the authorization though). This condition will be ignored for brands which Saferpay does not offer 3d-secure for. --- If left out, the authorization will be done if allowed, but possibly without liability shift to the issuer. See the specific result codes in the response message. Example: NONE
|
RegisterAlias object |
Controls whether the given means of payment should be stored inside the Saferpay Secure Card Data storage.
|
RequestHeader mandatory, object |
General information about the request.
|
Token mandatory, string |
Token returned by Initialize
Id[1..50]Example: 234uhfh78234hlasdfh8234e
|
VerificationCode string |
Card verification code if available
Numeric[3..4]Example: 123
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "Token": "sdu5ymxx210y2dz1ggig2ey0o", "VerificationCode": "123" }
Response
Arguments | |
---|---|
Dcc object |
Dcc information, if applicable
|
FraudPrevention object |
Contains details of a performed fraud prevention check
|
Liability object |
LiabilityShift information, replaces ThreeDs Info from API version 1.8
|
MastercardIssuerInstallments object |
Mastercard card issuer installment payment options, if applicable
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
RegistrationResult object |
Information about the Secure Card Data registration outcome.
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "MUOGAWA9pKr6rAv5dUKIbAjrCGYA", "Date": "2015-09-18T09:19:27.078Z", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "AcquirerName", "AcquirerReference": "Reference", "SixTransactionReference": "0:0:3:MUOGAWA9pKr6rAv5dUKIbAjrCGYA", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } }, "Payer": { "IpAddress": "1.2.3.4", "IpLocation": "DE" }, "Liability": { "LiabilityShift": true, "LiableEntity": "THREEDS", "ThreeDs": { "Authenticated": true, "Xid": "ARkvCgk5Y1t/BDFFXkUPGX9DUgs=" } } }
Transaction AuthorizeDirect Available depending on license
POST
This function may be used to directly authorize transactions which do not require a redirect of the customer (e.g. direct debit or recurring transactions based on a previously registered alias).
Warning: Only PCI certified merchants may submit the card-data directly, or use their own HTML form! Click here for more information!
Important: This function does not perform 3D Secure! Only the PaymentPage or Transaction Initialize do support 3D Secure!
Request URL:
POST: /Payment/v1/Transaction/AuthorizeDirect
Request
Arguments | |
---|---|
Authentication object |
Strong Customer Authentication exemption for this transaction.
Some exemptions are only applicable to payer-initiated transactions and will be ignored otherwise. If you are performing a payer-initiated transaction, make sure you set the 'Initiator' attribute properly (see below). |
Initiator string |
Specify if the transaction was initiated by the merchant (default behavior if not specified) or by the payer.
Possible values: MERCHANT, PAYER.This is relevant for most credit and debit cards managed by Mastercard, Visa and American Express card schemes (in card scheme jargon: MERCHANT means MIT, PAYER means CIT). For these schemes, transactions initiated by the payer usually require authentication of the card holder, which is not possible if you use Transaction/AuthorizeDirect (use Transaction/Initialize or PaymentPage/Initialize if you're not sure). Saferpay will flag the transaction accordingly (also taking the optional Exemption in the Authentication container into account) on the protocols which support this and card issuers might approve or decline transactions depending on this flagging. Example: MERCHANT
|
Order object |
Optional order information. Only used for payment method Klarna (mandatory) and for Fraud Intelligence (optional).
|
Payer object |
Information on the payer (IP-address)
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
PaymentMeans mandatory, object |
Information on the means of payment. Important: Only fully PCI certified merchants may directly use the card data. If your system is not explicitly certified to handle card data directly, then use the Saferpay Secure Card Data-Storage instead. If the customer enters a new card, you may want to use the Saferpay Hosted Register Form to capture the card data through Saferpay.
|
RegisterAlias object |
Controls whether the given means of payment should be stored inside the Saferpay Secure Card Data storage.
|
RequestHeader mandatory, object |
General information about the request.
|
RiskFactors object |
Optional risk factors
|
TerminalId mandatory, string |
Saferpay Terminal-Id
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "Description": "Test123", "PayerNote": "Order123_Testshop" }, "PaymentMeans": { "Card": { "Number": "912345678901234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "VerificationCode": "123" } }, "Authentication": { "Exemption": "RECURRING" } }
Response
Arguments | |
---|---|
FraudPrevention object |
Contains details of a performed fraud prevention check
|
Liability object |
LiabilityShift information, replaces ThreeDs Info from api version 1.26
|
MastercardIssuerInstallments object |
Mastercard card issuer installment payment options, if applicable
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
RegistrationResult object |
Information about the Secure Card Data registration outcome.
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "AcquirerName", "AcquirerReference": "Reference", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 7, "HolderName": "Max Mustermann", "CountryCode": "CH" } }, "Payer": { "IpAddress": "1.2.3.4", "IpLocation": "DE" } }
Transaction AuthorizeReferenced Available depending on license
POST
This method may be used to perform follow-up authorizations to an earlier transaction. At this time, the referenced (initial) transaction must have been performed setting either the recurring or installment option.
Request URL:
POST: /Payment/v1/Transaction/AuthorizeReferenced
Request
Arguments | |
---|---|
Authentication object |
Strong Customer Authentication (exemptions, ...)
|
Notification object |
Notification options
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
RequestHeader mandatory, object |
General information about the request.
|
SuppressDcc boolean |
Used to suppress direct currency conversion
|
TerminalId mandatory, string |
Saferpay Terminal-Id
Numeric[8..8]Example: 12341234
|
TransactionReference mandatory, object |
Reference to previous transaction.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "Description": "Test123", "PayerNote": "Order123_Testshop" }, "Authentication": { "Exemption": "RECURRING" }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
Dcc object |
Dcc information, if applicable
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "AcquirerName", "AcquirerReference": "Reference", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 7, "HolderName": "Max Mustermann", "CountryCode": "CH" } }, "Payer": { "IpAddress": "1.2.3.4", "IpLocation": "DE" }, "Dcc": { "PayerAmount": { "Value": "109", "CurrencyCode": "USD" } } }
Transaction Capture Available depending on license
POST
This method may be used to finalize previously authorized transactions and refunds.
Request URL:
POST: /Payment/v1/Transaction/Capture
Request
Arguments | |
---|---|
Amount object |
Currency must match the original transaction currency (request will be declined if currency does not match)
|
Billpay object |
Optional Billpay specific options.
|
Marketplace object |
Optional Marketplace capture parameters.
|
MastercardIssuerInstallments object |
Selected Mastercard card issuer installment payment option, if applicable
|
PendingNotification object |
Optional pending notification capture options for Paydirekt transactions.
|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to authorization.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
CaptureId string |
CaptureId of the created capture. Must be stored for later reference (eg refund).
Id[1..64]Example: ECthWpbv1SI6SAIdU2p6AIC1bppA_c
|
Date mandatory, date |
Date and time of capture. Not set if the capture state is pending.
Example: 2014-04-25T08:33:44.159+01:00
|
Invoice object |
Optional infos for invoice based payments.
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Status mandatory, string |
Current status of the capture. (PENDING is only used for paydirekt at the moment)
Possible values: PENDING, CAPTURED. |
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "CaptureId": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Status": "CAPTURED", "Date": "2015-01-30T12:45:22.258+01:00" }
Transaction MultipartCapture Available depending on license
POST
This method may be used to capture multiple parts of an authorized transaction.
Important:
- MultipartCapture is available for PayPal, Klarna and card payments Visa, Mastercard, Maestro, Diners/Discover, JCB and American Express which are acquired by Worldline.
- No MultipartCapture request should be sent before receiving the response of a preceding request (i.e. no parallel calls are allowed).
- The sum of multipart captures must not exceed the authorized amount.
- A unique OrderPartId must be used for each request.
Request URL:
POST: /Payment/v1/Transaction/MultipartCapture
Request
Arguments | |
---|---|
Amount mandatory, object |
Currency must match the original transaction currency (request will be declined if currency does not match)
|
Marketplace object |
Optional Marketplace capture parameters.
|
OrderPartId mandatory, string |
Must be unique. It identifies each individual step and is especially important for follow-up actions such as refund.
Id[1..80]Example: kh9ngajrfe6wfu3d0c
|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to authorization.
Exactly one element must be set. |
Type mandatory, string |
'PARTIAL' if more captures should be possible later on, 'FINAL' if no more captures will be done on this authorization.
Possible values: PARTIAL, FINAL. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" }, "Amount": { "Value": "1000", "CurrencyCode": "CHF" }, "Type": "PARTIAL", "OrderPartId": "123456789", "Marketplace": { "SubmerchantId": "17312345", "Fee": { "Value": "500", "CurrencyCode": "CHF" } } }
Response
Arguments | |
---|---|
CaptureId string |
CaptureId of the created capture. Must be stored for later reference (eg refund).
Id[1..64]Example: ECthWpbv1SI6SAIdU2p6AIC1bppA_c
|
Date mandatory, date |
Date and time of capture. Not set if the capture state is pending.
Example: 2018-08-08T12:45:22.258+01:00
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Status mandatory, string |
Current status of the capture. (PENDING is only used for paydirekt at the moment)
Possible values: PENDING, CAPTURED. |
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[unique request identifier]" }, "CaptureId": "723n4MAjMdhjSAhAKEUdA8jtl9jb_c", "Status": "CAPTURED", "Date": "2018-08-08T13:45:22.258+02:00" }
Transaction AssertCapture Available depending on license
POST
Attention: This method is only supported for pending captures. A pending capture is only applicable for paydirekt transactions at the moment.
Request URL:
POST: /Payment/v1/Transaction/AssertCapture
Request
Arguments | |
---|---|
CaptureReference mandatory, object |
Reference to the capture.
|
RequestHeader mandatory, object |
General information about the request.
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "CaptureReference": { "CaptureId": "24218eabae254caea6f898e413fe_c" } }
Response
Arguments | |
---|---|
Date mandatory, date |
Date and time of capture. Not set if the capture state is pending.
Example: 2014-04-25T08:33:44.159+01:00
|
OrderId string |
OrderId of the referenced transaction. If present.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Status mandatory, string |
Current status of the capture. (PENDING is only used for paydirekt at the moment)
Possible values: PENDING, CAPTURED. |
TransactionId mandatory, string |
Id of the referenced transaction.
AlphaNumeric[1..64]Example: 723n4MAjMdhjSAhAKEUdA8jtl9jb
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Status": "CAPTURED", "Date": "2015-01-30T12:45:22.258+01:00" }
Transaction MultipartFinalize Available depending on license
POST
This method may be used to finalize a transaction having one or more partial captures (i.e. marks the end of partial captures).
Request URL:
POST: /Payment/v1/Transaction/MultipartFinalize
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to authorization.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[unique request identifier]" } }
Transaction Refund Available depending on license
POST
This method may be called to refund a previous transaction.
Request URL:
POST: /Payment/v1/Transaction/Refund
Request
Arguments | |
---|---|
CaptureReference mandatory, object |
Reference to the capture you want to refund.
|
PaymentMethodsOptions object |
|
PendingNotification object |
Optional pending notification options
|
Refund mandatory, object |
Information about the refund (amount, currency, ...)
|
RequestHeader mandatory, object |
General information about the request.
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "Refund": { "Amount": { "Value": "100", "CurrencyCode": "CHF" } }, "CaptureReference": { "CaptureId": "723n4MAjMdhjSAhAKEUdA8jtl9jb_c" } }
Response
Arguments | |
---|---|
Dcc object |
Dcc information, if applicable
|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "REFUND", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test", "AcquirerReference": "000000", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Transaction AssertRefund Available depending on license
POST
This method may be used to inquire the status and further information of pending refunds.
Attention: This method is only supported for pending refunds. A pending refund is only applicable for paydirekt or WL Crypto Payments transactions at the moment.
Request URL:
POST: /Payment/v1/Transaction/AssertRefund
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to authorization.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
Date mandatory, date |
Date and time of capture. Not set if the capture state is pending.
Example: 2014-04-25T08:33:44.159+01:00
|
OrderId string |
OrderId of the referenced transaction. If present.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Status mandatory, string |
Current status of the capture. (PENDING is only used for paydirekt at the moment)
Possible values: PENDING, CAPTURED. |
TransactionId mandatory, string |
Id of the referenced transaction.
AlphaNumeric[1..64]Example: 723n4MAjMdhjSAhAKEUdA8jtl9jb
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Status": "CAPTURED", "Date": "2015-01-30T12:45:22.258+01:00" }
Transaction RefundDirect Available depending on license
POST
This method may be called to refund an amount to the given means of payment (not supported for all means of payment) without referencing a previous transaction. This might be the case if the original transaction was done with a card which is not valid any more.
Warning: Only PCI certified merchants may submit the card-data directly, or use their own HTML form! Click here for more information!
Request URL:
POST: /Payment/v1/Transaction/RefundDirect
Request
Arguments | |
---|---|
OriginalCreditTransfer object |
Information about the Original Credit Transfer like the Address of the Recipient.
|
PaymentMeans mandatory, object |
Information on the means of payment. Important: Only fully PCI certified merchants may directly use the card data.
If your system is not explicitly certified to handle card data directly, then use the Saferpay Secure Card Data-Storage instead. If the customer enters a new card, you may want to use the Saferpay Hosted Register Form to capture the card data through Saferpay. |
Refund mandatory, object |
Information about the refund (amount, currency, ...)
|
RequestHeader mandatory, object |
General information about the request.
|
TerminalId mandatory, string |
Saferpay Terminal-Id
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Refund": { "Amount": { "Value": "100", "CurrencyCode": "CHF" } }, "PaymentMeans": { "Alias": { "Id": "alias35nfd9mkzfw0x57iwx" } } }
Response
Arguments | |
---|---|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "REFUND", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test", "AcquirerReference": "000000", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Transaction Cancel Available depending on license
POST
This method may be used to cancel previously authorized transactions and refunds.
Request URL:
POST: /Payment/v1/Transaction/Cancel
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to transaction to be canceled.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
Date mandatory, date |
Date and time of cancel.
Example: 2014-04-25T08:33:44.159+01:00
|
OrderId string |
OrderId of the referenced transaction. If present.
Example: c52ad18472354511ab2c33b59e796901
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
TransactionId mandatory, string |
Id of the referenced transaction.
Example: qiuwerhfi23h4189asdhflk23489
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "OrderId": "c52ad18472354511ab2c33b59e796901", "Date": "2015-01-30T12:45:22.258+01:00" }
Transaction RedirectPayment Available depending on license
POST
WARNING: This feature is deprecated and replaced by the Payment Page. Please use the parameter PaymentMethods to directly select the desired 3rd party provider!
Request URL:
POST: /Payment/v1/Transaction/RedirectPayment
Request
Arguments | |
---|---|
Notification object |
Notification options
|
Payer object |
Information on the payer (IP-address)
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
RequestHeader mandatory, object |
General information about the request.
|
ReturnUrl mandatory, object |
URL which is used to redirect the payer back to the shop if the transaction requires some kind of browser redirection (3d-secure, dcc)
This Url is used by Saferpay to redirect the shopper back to the merchant shop. You may add query string parameters to identify your session, but please be aware that the shopper could modify these parameters inside the browser! The whole url including query string parameters should be as short as possible to prevent issues with specific browsers and must not exceed 2000 characters. Note: you should not add sensitive data to the query string, as its contents is plainly visible inside the browser and will be logged by our web servers. |
ServiceProvider mandatory, string |
Service provider to be used for this payment
Possible values: POSTCARD, POSTFINANCE.Example: POSTFINANCE
|
Styling object |
Custom styling resource
|
TerminalId mandatory, string |
Saferpay terminal to use for this authorization
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" } }, "ServiceProvider": "POSTFINANCE", "ReturnUrl": { "Url": "[your shop payment url]" } }
Response
Arguments | |
---|---|
Expiration mandatory, date |
Expiration date / time of the generated token in ISO 8601 format in UTC. After this time, the token won’t be accepted for any further action.
Example: 2015-01-30T13:45:22.258+02:00
|
RedirectUrl string |
Url to redirect the browser to for payment processing
Example: https://www.saferpay.com/VT2/api/...
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Token mandatory, string |
Id for referencing later
Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Token": "234uhfh78234hlasdfh8234e", "Expiration": "2015-01-30T12:45:22.258+01:00", "RedirectUrl": "https://www.saferpay.com/vt2/Api/..." }
Transaction AssertRedirectPayment Available depending on license
POST
WARNING: This feature is deprecated and replaced by the Payment Page. Please use the parameter PaymentMethods to directly select the desired 3rd party provider!
Request URL:
POST: /Payment/v1/Transaction/AssertRedirectPayment
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
Token mandatory, string |
Token returned by initial call.
Id[1..50]Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "Token": "234uhfh78234hlasdfh8234e" }
Response
Arguments | |
---|---|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test", "AcquirerReference": "8EZRQVT0ODW4ME525", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "Number": "912345678901234", "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2022, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Transaction Inquire Available depending on license
POST
This method can be used to get the details of a transaction that has been authorized successfully.
Fair use:This method is not intended for polling. You have to restrict the usage of this method in order to provide a fair data access to all our customers. We may contact you if we notice the excessive usage of this function and in some exceptional cases we preserve the right to limit the access to it.
Request URL:
POST: /Payment/v1/Transaction/Inquire
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
TransactionReference mandatory, object |
Reference to authorization.
Exactly one element must be set. |
Example:
{ "RequestHeader": { "SpecVersion": "1.11", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TransactionReference": { "TransactionId": "723n4MAjMdhjSAhAKEUdA8jtl9jb" } }
Response
Arguments | |
---|---|
Dcc object |
Dcc information, if applicable
|
FraudPrevention object |
Contains details of a performed fraud prevention check
|
Liability object |
LiabilityShift information, replaces ThreeDs Info from api version 1.8
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "1.11", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "CAPTURED", "Id": "UlKpE6A2UxlttAOQtnYIbCj1CpIA", "Date": "2019-04-05T10:49:55.76+02:00", "Amount": { "Value": "1200", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test Card", "AcquirerReference": "32436794662", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA" }, "DisplayText": "xxxx xxxx xxxx 0004", "Card": { "MaskedNumber": "901050xxxxxx0004", "ExpYear": 2022, "ExpMonth": 6, "HolderName": "Max Mustermann", "CountryCode": "CH" } }, "Liability": { "LiabilityShift": true, "LiableEntity": "THREEDS", "ThreeDs": { "Authenticated": true, "Xid": "ARkvCgk5Y1t/BDFFXkUPGX9DUgs=" } } }
Transaction AlternativePayment Available depending on license
POST
This method can be used to authorize the payments that do not have a payment-page or
for the payments that before authorization some additional steps such as authentication should be done.
Request URL:
POST: /Payment/v1/Transaction/AlternativePayment
Request
Arguments | |
---|---|
Notification mandatory, object |
Notification options
|
Order object |
Optional order information. Only used for payment method Klarna (mandatory) and for Fraud Intelligence (optional).
|
Payer object |
Information on the payer (IP-address)
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
PaymentMethod mandatory, string |
Service provider to be used for this payment
Possible values: BANCONTACT.Example: BANCONTACT
|
PaymentMethodOptions object |
Optional. May be used to set specific options for the payment method.
|
RequestHeader mandatory, object |
General information about the request.
|
RiskFactors object |
Optional risk factors
|
TerminalId mandatory, string |
Saferpay terminal to use for this authorization
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "1.12", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" } }, "PaymentMethod": "BANCONTACT" }
Response
Arguments | |
---|---|
Expiration mandatory, date |
Expiration date / time of the generated token in ISO 8601 format in UTC. After this time, the token won’t be accepted for any further action.
Example: 2015-01-30T13:45:22.258+02:00
|
ProcessingData object |
data required by the merchant system to process the payment (e.g. QR-code data, intent URL, ...)
Payment method specific data required to process an alternative payment. Only one container (matching the PaymentMethod of the AlternativePaymentRequest message) will be present |
ResponseHeader mandatory, object |
Contains general information about the response.
|
Token mandatory, string |
Id for referencing later
Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Token": "234uhfh78234hlasdfh8234e", "Expiration": "2015-01-30T12:45:22.258+01:00", "ProcessingData": { "Bancontact": { "QrCodeData": "someQRcodeData", "IntentUrl": "https://www.saferpay.com/vt2/Api/..." } } }
Transaction QueryAlternativePayment Available depending on license
POST
Call this method to get information about a previously initialized alternative payment transaction
Request URL:
POST: /Payment/v1/Transaction/QueryAlternativePayment
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
Token mandatory, string |
Token returned by initial call.
Id[1..50]Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "Token": "234uhfh78234hlasdfh8234e" }
Response
Arguments | |
---|---|
FraudPrevention object |
Contains details of a performed fraud prevention check
|
Liability object |
LiabilityShift information, replaces ThreeDs Info from api version 1.8
|
Payer object |
Information about the payer / card holder
|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "1.12", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "CAPTURED", "Id": "ChAChMA5hx89vAAzEh52AUYvxWCb", "CaptureId": "ChAChMA5hx89vAAzEh52AUYvxWCb", "Date": "2019-06-19T15:04:48.733+02:00", "Amount": { "Value": "100", "CurrencyCode": "EUR" }, "AcquirerName": "Bancontact Saferpay Test", "AcquirerReference": "32332251189", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "945011" }, "PaymentMeans": { "Brand": { "PaymentMethod": "BANCONTACT", "Name": "Bancontact" }, "DisplayText": "xxxx xxxx xxxx x000 5", "Card": { "MaskedNumber": "xxxxxxxxxxxxx0005", "ExpYear": 2020, "ExpMonth": 6, "CountryCode": "BE" } }, "Liability": { "LiabilityShift": true, "LiableEntity": "THREEDS", "ThreeDs": { "Authenticated": true, "Xid": "ARkvCgk5Y1t/BDFFXkUPGX9DUgs=" } } }
Secure Card Data
Alias Insert
POST
This function may be used to insert an alias without knowledge about the card details. Therefore a redirect of the customer is required.
Warning: Only PCI certified merchants may submit the card-data directly, or use their own HTML form! Click here for more information!
Request URL:
POST: /Payment/v1/Alias/Insert
Request
Arguments | |
---|---|
CardForm object |
Options for card data entry form (if applicable)
|
Check object |
Parameters for checking the means of payment before registering.
IMPORTANT NOTE: The Check function is only available for certain brands / acquirers! For more information, see Saferpay Integration Guide for Secure Card Data |
LanguageCode string |
Language used for displaying forms. Format: ISO 639-1 (two-letter language code), optionally followed by a hyphen and ISO 3166-1 alpha-2 (two-letter country code).
Example: The supported language codes are listed below. This list may be extended in the future as more languages become available. We recommend to only use supported language and country codes. If the submitted value has a valid format, but the language is unsupported, then the default language is used. For supported languages, using different country codes than those explicitly listed here may or may not work as expected. Code-List: bg - Bulgarian cs - Czech da - Danish de - German de-CH - Swiss German el - Greek en - English es - Spanish et - Estonian fi - Finnish fr - French hr - Croatian hu - Hungarian is - Icelandic it - Italian ja - Japanese lt - Lithuanian lv - Latvian nl - Dutch nn - Norwegian pl - Polish pt - Portuguese ro - Romanian ru - Russian sk - Slovak sl - Slovenian sv - Swedish tr - Turkish uk - Ukrainian zh - Chinese de
|
Notification object |
Url to which Saferpay will send the asynchronous confirmation for the process completion. Supported schemes are http and https. You also have to make sure to support the GET-method.
Example: https://merchanthost/notify/123
|
PaymentMeans object |
Means of payment to register
|
PaymentMethods array of strings |
Used to restrict the means of payment which are available to the payer
Possible values: AMEX, BONUS, DINERS, DIRECTDEBIT, JCB, MAESTRO, MASTERCARD, MYONE, VISA. Additional values may be accepted but are ignored.Example: ["VISA", "MASTERCARD"]
|
RegisterAlias mandatory, object |
Registration parameters
|
RequestHeader mandatory, object |
General information about the request.
|
ReturnUrl mandatory, object |
URL which is used to redirect the payer back to the shop.
This Url is used by Saferpay to redirect the shopper back to the merchant shop. You may add query string parameters to identify your session, but please be aware that the shopper could modify these parameters inside the browser! The whole url including query string parameters should be as short as possible to prevent issues with specific browsers and must not exceed 2000 characters. Note: you should not add sensitive data to the query string, as its contents is plainly visible inside the browser and will be logged by our web servers. |
Styling object |
Custom styling resource for the Hosted Register form.
|
Type mandatory, string |
Type of payment means to register
Possible values: CARD, BANK_ACCOUNT, POSTFINANCEPAY, TWINT.Example: CARD
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "RegisterAlias": { "IdGenerator": "RANDOM" }, "Type": "CARD", "ReturnUrl": { "Url": "[your shop alias registration url]" } }
Response
Arguments | |
---|---|
Expiration mandatory, date |
Expiration date / time of the generated token in ISO 8601 format in UTC.
Example: After this time is exceeded, the token will not be accepted for any further actions except Asserts. 2015-01-30T13:45:22.258+02:00
|
Redirect object |
Mandatory if RedirectRequired is true. Contains the URL for the redirect to use for example the Saferpay hosted register form.
|
RedirectRequired boolean |
True if a redirect must be performed to continue, false otherwise
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Token mandatory, string |
Id for referencing later
Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Token": "234uhfh78234hlasdfh8234e", "Expiration": "2015-01-30T12:45:22.258+01:00", "RedirectRequired": true, "Redirect": { "RedirectUrl": "https://www.saferpay.com/VT2/api/...", "PaymentMeansRequired": false } }
Alias AssertInsert
POST
This method may be used to inquire the Alias Id and further information after a successful Alias Insert call.
This function can be called up to 24 hours after the transaction was initialized.
Caution: Please DO NOT use the AssertInsert request for polling. You should always await the reception of the SuccessUrl.
Request URL:
POST: /Payment/v1/Alias/AssertInsert
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
Token mandatory, string |
Token returned by initial call.
Id[1..50]Example: 234uhfh78234hlasdfh8234e
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request identifier]", "RetryIndicator": 0 }, "Token": "234uhfh78234hlasdfh8234e" }
Response
Arguments | |
---|---|
Alias mandatory, object |
Information about the registered alias.
|
CheckResult object |
Result of the Check
|
PaymentMeans mandatory, object |
Information about the registered means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Alias": { "Id": "alias35nfd9mkzfw0x57iwx", "Lifetime": 1000 }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Alias InsertDirect
POST
This method may be used to insert an alias directly with card-data collected by using your own HTML form.
Warning: Only respectively PCI certified merchants may submit the card-data directly, or use their own HTML form! Click here for more information!
Request URL:
POST: /Payment/v1/Alias/InsertDirect
Request
Arguments | |
---|---|
Check object |
Parameters for checking the means of payment before registering. IMPORTANT NOTE: The Check function is only available for SIX Payment Services VISA and Mastercard acquiring contracts!
|
IssuerReference object |
Contains that is received from the issuer in the response of a successful payment by other payment providers. This data will be used for authorizations based on this alias.
|
PaymentMeans mandatory, object |
Means of payment to register
|
RegisterAlias mandatory, object |
Registration parameters
|
RequestHeader mandatory, object |
General information about the request.
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "PaymentMeans": { "Card": { "Number": "912345678901234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "VerificationCode": "123" } }, "RegisterAlias": { "IdGenerator": "RANDOM" } }
Response
Arguments | |
---|---|
Alias mandatory, object |
Information about the registered alias.
|
CheckResult object |
Result of the Check
|
PaymentMeans mandatory, object |
Information about the registered means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Alias": { "Id": "alias35nfd9mkzfw0x57iwx", "Lifetime": 1000 }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Alias Update
POST
This method may be used to update an alias' lifetime and / or its credit card expiry date
Request URL:
POST: /Payment/v1/Alias/Update
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
UpdateAlias mandatory, object |
Update parameters
|
UpdatePaymentMeans mandatory, object |
Means of payment to update
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "AliasUpdate": { "Id": "[your alias id]", "Lifetime": "[your lifetime]" }, "UpdatePaymentMeans": { "Card": { "ExpMonth": "[your expiry month]", "ExpYear": "[your expiry year]" } } }
Response
Arguments | |
---|---|
Alias mandatory, object |
Information about the registered alias.
|
PaymentMeans mandatory, object |
Information about the registered means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Alias": { "Id": "alias35nfd9mkzfw0x57iwx", "Lifetime": 1000 }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Alias Delete
POST
This method may be used to delete previously inserted aliases.
Request URL:
POST: /Payment/v1/Alias/Delete
Request
Arguments | |
---|---|
AliasId mandatory, string |
The Alias you want to delete. This value is case-insensitive.
Id[1..40]Example: alias35nfd9mkzfw0x57iwx
|
RequestHeader mandatory, object |
General information about the request.
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "AliasId": "alias35nfd9mkzfw0x57iwx" }
Response
Arguments | |
---|---|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" } }
Batch
Batch Close
POST
This chapter covers the Batch Close via API
Note: If you want to trigger the batch-close via API, make sure, to turn the automatic batch off. Please log into the Saferpay Backoffice. Go to Settings => Terminals and select the desired terminal out of the list. Turn off the Automatic closure.
Request URL:
POST: /Payment/v1/Batch/Close
Request
Arguments | |
---|---|
RequestHeader mandatory, object |
General information about the request.
|
TerminalId mandatory, string |
Saferpay Terminal-Id
Numeric[8..8]Example: 12341234
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[unique request id]", "RetryIndicator": 0 }, "TerminalId": "[your terminal id]" }
Response
Arguments | |
---|---|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" } }
OmniChannel
OmniChannel InsertAlias
POST
This function may be used to create an alias by providing a SixTransactionReference.
Request URL:
POST: /Payment/v1/OmniChannel/InsertAlias
Request
Arguments | |
---|---|
RegisterAlias mandatory, object |
Registration parameters
|
RequestHeader mandatory, object |
General information about the request.
|
SixTransactionReference mandatory, string |
SIX Transaction Reference
Example: 1:100002:199970683910
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "SixTransactionReference": "1:100002:1:87768996410", "RegisterAlias": { "IdGenerator": "RANDOM" } }
Response
Arguments | |
---|---|
Alias mandatory, object |
Information about the registered alias.
|
PaymentMeans mandatory, object |
Information about the registered means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Alias": { "Id": "alias35nfd9mkzfw0x57iwx", "Lifetime": 1000 }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
OmniChannel AcquireTransaction
POST
This function may be used to acquire a POS authorized or captured transaction by providing a SixTransactionReference. This transaction can then be authorized, canceled, captured or refunded.
Request URL:
POST: /Payment/v1/OmniChannel/AcquireTransaction
Request
This request allows to acquire an OmniChannel transaction.
Arguments | |
---|---|
OrderId string |
Unambiguous order identifier defined by the merchant/ shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
RequestHeader mandatory, object |
General information about the request.
|
SixTransactionReference mandatory, string |
SIX Transaction Reference
Example: 1:100002:199970683910
|
TerminalId mandatory, string |
Saferpay terminal id
Numeric[8..8]Example: 12345678
|
Example:
{ "RequestHeader": { "SpecVersion": "[current Spec-Version]", "CustomerId": "[your customer id]", "RequestId": "[your request id]", "RetryIndicator": 0 }, "TerminalId": "17791723", "SixTransactionReference": "1:100002:1:87768996410", "OrderId": "Id of the order" }
Response
This response returns an acquired OmniChannel transaction.
Arguments | |
---|---|
PaymentMeans mandatory, object |
Information about the means of payment
|
ResponseHeader mandatory, object |
Contains general information about the response.
|
Transaction mandatory, object |
Information about the transaction
|
Example:
{ "ResponseHeader": { "SpecVersion": "[current Spec-Version]", "RequestId": "[your request id]" }, "Transaction": { "Type": "PAYMENT", "Status": "AUTHORIZED", "Id": "723n4MAjMdhjSAhAKEUdA8jtl9jb", "Date": "2015-01-30T12:45:22.258+01:00", "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "AcquirerName": "Saferpay Test", "AcquirerReference": "8EZRQVT0ODW4ME525", "SixTransactionReference": "0:0:3:723n4MAjMdhjSAhAKEUdA8jtl9jb", "ApprovalCode": "012345" }, "PaymentMeans": { "Brand": { "PaymentMethod": "VISA", "Name": "VISA Saferpay Test" }, "DisplayText": "9123 45xx xxxx 1234", "Card": { "Number": "912345678901234", "MaskedNumber": "912345xxxxxx1234", "ExpYear": 2015, "ExpMonth": 9, "HolderName": "Max Mustermann", "CountryCode": "CH" } } }
Management API
This interface offers REST based access to various management features. This enables customers to integrate those features into their systems on a technical level.
Important Note: The Saferpay Management API does not use the JSON API header. Instead, some additional HTTP header fields are required.
Additional HTTP Headers
Instead of the RequestHeader
container used in the JSON API, the Management API uses additional HTTP Headers to store and transfer general information about the request.
All other requirements regarding the HTTP Headers (e.g. for content encoding and authentication) still apply.
Header field | Possible values | Description |
---|---|---|
Saferpay-ApiVersion | 1.14 | Version number of the interface specification. |
Saferpay-RequestId | Id[1..50] | Id generated by merchant system, for debugging purposes. Should be unique for each new request. If a request is retried due to an error, use the same request id. |
HTTP Headers example:
Saferpay-ApiVersion: 1.14
Saferpay-RequestId: 33e8af17-35c1-4165-a343-c1c86a320f3b
Licensing CustomerLicense
GET
This method is used to retrieve the current license configuration for a customer
Request URL:
GET: /rest/customers/[customerId]/license
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
Features mandatory, array of objects |
All features contained in the configured package or assigned additionally
|
Package mandatory, object |
Information about the configured package
|
Example:
{ "Package": { "Id": "SAFERPAY_FLEX", "DisplayName": "Saferpay Flex" }, "Features": [ { "Id": "MANAGEMENT_API", "DisplayName": "Management API" }, { "Id": "PAYMENT_API", "DisplayName": "Payment API" } ] }
Licensing CustomerLicenseConfiguration
GET
DEPRECATED: since Version 1.35. Please use instead: /rest/customers/{customerId}/license
This method is used to retrieve the current license configuration for a customer
Request URL:
GET: /rest/customers/[customerId]/license-configuration
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
Features mandatory, array of objects |
All features contained in the configured package or assigned additionally
|
Package mandatory, object |
Information about the configured package
|
Example:
{ "Package": { "Id": "SAFERPAY_FLEX", "DisplayName": "Saferpay Flex" }, "Features": [ { "Id": "MANAGEMENT_API", "DisplayName": "Management API" }, { "Id": "PAYMENT_API", "DisplayName": "Payment API" } ] }
SaferpayFieldsAccessToken CreateAccessToken
POST
Create a Saferpay Fields Access Token that can be used to integrate Saferpay Fields into web pages and is restricted to the given customerId, terminalId and URL(s).
Request URL:
POST: /rest/customers/[customerId]/terminals/[terminalId]/fields-access-tokens
Request
Arguments | |
---|---|
Description mandatory, string |
A human readable description that will be shown in the Saferpay Backoffice to easily identify the Access Token.
Utf8[1..128] |
SourceUrls mandatory, string[] |
The fully qualified URL of the shop (the page that integrates Saferpay Fields). We recommend that URLs end with a trailing slash. Only HTTPS is allowed.
Example: https://yourshop.com/
|
Example:
{ "SourceUrls": [ "https://a.yourshop.com/", "https://b.yourshop.com/" ], "Description": "Saferpay Fields Access Token for YourShop" }
Response
Arguments | |
---|---|
AccessToken mandatory, string |
The AccessToken for the Saferpay Fields JavaScript library
Example: 67f47961-2912-4338-8039-22ac2b8486f3
|
Example:
{ "AccessToken": "67f47961-2912-4338-8039-22ac2b8486f3" }
SecurePayGate Create SingleUsePaymentLink
POST
This function may be used to create a single use payment link
Request URL:
POST: /rest/customers/[customerId]/terminals/[terminalId]/spg-offers
Request
Arguments | |
---|---|
BillingAddressForm object |
Used to have the payer enter or change his billing address in the payment process.
|
Condition string |
THREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED: the authorization will be executed if the previous 3d-secure process indicates that the liability shift to the issuer is possible
Possible values: NONE, THREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED, WITH_SUCCESSFUL_THREE_DS_CHALLENGE.(liability shift may still be declined with the authorization though). This condition will be ignored for brands which Saferpay does not offer 3d-secure for. WITH_SUCCESSFUL_THREE_DS_CHALLENGE: Will force the 3D-Secure challenge. --- If left out, the authorization will be done if allowed, but possibly without liability shift to the issuer. See the specific result codes in the response message. Example: NONE
|
ConfigSet string |
This parameter lets you define your payment page config (PPConfig) by name. If this parameter is not set, your default PPConfig will be applied if available.
Id[1..20]When the PPConfig can't be found (e.g. wrong name), the Saferpay basic style will be applied to the payment page. Example: name of your payment page config (case-insensitive)
|
ExpirationDate mandatory, string |
The date after which the offer expires in ISO 8601 format.
AlphaNumeric[10..25]yyyy-MM-ddTHH:mm:ssK Must be at least 1h in the future and within the next 180 days. Example: 2019-10-20T00:00:00+02:00
|
Order object |
Optional order information. Only used for payment method Klarna (mandatory) and for Fraud Intelligence (optional).
|
Payer mandatory, object |
Information about the payer
|
Payment mandatory, object |
Information about the payment (amount, currency, ...)
|
RegisterAlias object |
Controls whether the means of payment used for paying the offer should be stored inside the Saferpay Secure Card Data storage.
If the offer is paid using a payment means that does not support being stored in the Secure Card Data storage, this parameter has no effect. |
Example:
{ "Payment": { "Amount": { "Value": "404", "CurrencyCode": "CHF" }, "OrderId": "094c2a7ce1374f7ca184591f123b154d", "Options": { "PreAuth": true } }, "ExpirationDate": "2020-04-23T00:00:00+02:00", "Payer": { "LanguageCode": "de", "BillingAddress": { "FirstName": "John", "LastName": "Doe", "Company": "Worldline", "Gender": "MALE", "Street": "Mustergasse 123", "Zip": "8008", "City": "Zurich", "CountryCode": "CH", "Email": "payer@provider.com" } } }
Response
Arguments | |
---|---|
OfferId mandatory, globally unique identifier |
The Id of the SecurePayGate offer
Example: 503a3d7b-072b-400f-9e7e-8ec15191c737
|
PaymentLink mandatory, string |
The SecurePayGate link for the payment
Example: https://www.saferpay.com/SecurePayGate/Payment/123456/12345678/503a3d7b-072b-400f-9e7e-8ec15191c737
|
Example:
{ "OfferId": "503a3d7b-072b-400f-9e7e-8ec15191c737", "PaymentLink": "https://www.saferpay.com/SecurePayGate/Payment/123456/12345678/503a3d7b-072b-400f-9e7e-8ec15191c737" }
SecurePayGate SingleUsePaymentLink
GET
This function may be used to fetch the status of a previously created single use payment link
Request URL:
GET: /rest/customers/[customerId]/terminals/[terminalId]/spg-offers/[offerId]
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
CreationDate date |
The creation date and time of the SecurePayGate offer
Example: 2023-02-24T11:34:11.128+01:00
|
ExpirationDate date |
The date after which the offer expires
Example: 2023-04-20T00:00:00.000+02:00
|
OfferId globally unique identifier |
The Id of the SecurePayGate offer
Example: 503a3d7b-072b-400f-9e7e-8ec15191c737
|
Payment object |
Information about the payment (amount, currency, description, orderId)
|
PaymentLink string |
The SecurePayGate link for the payment
Example: https://www.saferpay.com/SecurePayGate/Payment/123456/12345678/503a3d7b-072b-400f-9e7e-8ec15191c737
|
Status string |
The status of the SecurePayGate offer
Possible values: OPEN, PAID, EXPIRED.Example: PAID
|
TransactionId string |
The transaction id once a transaction was made
Example: vA3bhlAht2WlSAOKOvQSAOnbAdCb
|
Example:
{ "OfferId": "503a3d7b-072b-400f-9e7e-8ec15191c737", "PaymentLink": "https://www.saferpay.com/SecurePayGate/Payment/123456/12345678/503a3d7b-072b-400f-9e7e-8ec15191c737", "Status": "OPEN", "Payment": { "Amount": { "Value": "100", "CurrencyCode": "CHF" }, "OrderId": "cd0005bf21bb4906bcbea939d74cee72", "Description": "Hallo" }, "CreationDate": "2023-03-01T11:37:47.682+01:00", "ExpirationDate": "2023-04-01T00:00:00+02:00" }
DELETE
This function may be used to delete a single use payment link before it's expiry
Request URL:
DELETE: /rest/customers/[customerId]/terminals/[terminalId]/spg-offers/[offerId]
Request
No body needed
Example:
No example available
Response
Terminal GetTerminal
GET
This method is used to retrieve details of one terminal
Request URL:
GET: /rest/customers/[customerId]/terminals/[terminalId]
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
Description string |
Description of the terminal
|
PaymentMethods array of objects |
Array of payment methods that are available for the terminal
|
TerminalId string |
The Id of the terminal
|
Type string |
The type of the terminal
Possible values: ECOM, SPG, MPO. |
Wallets array of objects |
Array of wallets that are available for the terminal
|
Example:
{ "TerminalId": "1000001", "Type": "ECOM", "Description": "Terminal 1 for Payment Page / Ecommerce", "PaymentMethods": [ { "PaymentMethod": "VISA", "Currencies": [ "USD" ], "LogoUrl": "https://www.saferpay.com/static/logo/visa.svg?v=637381079349290910" }, { "PaymentMethod": "MASTERCARD", "Currencies": [ "EUR", "CHF" ], "LogoUrl": "https://www.saferpay.com/static/logo/mastercard.svg?v=637381079349290910" }, { "PaymentMethod": "MAESTRO", "Currencies": [ "EUR" ], "LogoUrl": "https://www.saferpay.com/static/logo/maestro.svg?v=637381079349290910" }, { "PaymentMethod": "PAYPAL", "Currencies": [ "EUR", "CHF", "USD" ], "LogoUrl": "https://www.saferpay.com/static/logo/paypal.svg?v=637381079349290910" } ], "Wallets": [ { "WalletName": "APPLEPAY", "LogoUrl": "https://www.saferpay.com/static/logo/applepay.svg?v=637381079349290910" }, { "WalletName": "GOOGLEPAY", "LogoUrl": "https://www.saferpay.com/static/logo/googlepay.svg?v=637381079349290910" } ] }
TerminalInfo PaymentMethods
GET
DEPRECATED:: since Version 1.31. Please use instead: /rest/customers/{customerId}/terminals/{terminalId}/
This method is used to retrieve a list of all available payment methods and wallets for a terminal.
Request URL:
GET: /rest/customers/[customerId]/terminals/[terminalId]/payment-methods
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
Wallets array of objects |
Array of wallets that are available for the terminal
|
PaymentMethods array of objects |
Array of payment methods that are available for the terminal
|
Example:
{ "PaymentMethods": [ { "PaymentMethod": "VISA", "Currencies": [ "USD" ], "LogoUrl": "https://www.saferpay.com/static/logo/visa.svg?v=637381079349290910" }, { "PaymentMethod": "MASTERCARD", "Currencies": [ "EUR", "CHF" ], "LogoUrl": "https://www.saferpay.com/static/logo/mastercard.svg?v=637381079349290910" }, { "PaymentMethod": "MAESTRO", "Currencies": [ "EUR" ], "LogoUrl": "https://www.saferpay.com/static/logo/maestro.svg?v=637381079349290910" }, { "PaymentMethod": "PAYPAL", "Currencies": [ "EUR", "CHF", "USD" ], "LogoUrl": "https://www.saferpay.com/static/logo/paypal.svg?v=637381079349290910" } ], "Wallets": [ { "WalletName": "APPLEPAY", "LogoUrl": "https://www.saferpay.com/static/logo/applepay.svg?v=637381079349290910" }, { "WalletName": "GOOGLEPAY", "LogoUrl": "https://www.saferpay.com/static/logo/googlepay.svg?v=637381079349290910" } ] }
Terminals GetTerminals
GET
This method is used to retrieve all terminals
Request URL:
GET: /rest/customers/[customerId]/terminals
Request
No body needed
Example:
No example available
Response
Arguments | |
---|---|
Terminals array of objects |
Array of terminals
|
Example:
{ "Terminals": [ { "TerminalId": "1000001", "Type": "ECOM", "Description": "Terminal 1 for Payment Page / Ecommerce" }, { "TerminalId": "1000002", "Type": "MPO", "Description": "Terminal 2 for Mail Phone Order" }, { "TerminalId": "1000002", "Type": "SPG", "Description": "Terminal 3 for Secure Pay Gate" } ] }
Container Dictionary
Container "Common_Models_Data_AmountWithoutZero"
CurrencyCode mandatory, string |
ISO 4217 3-letter currency code (CHF, USD, EUR, ...)
Example: CHF
|
Value mandatory, string |
Amount in minor unit (CHF 1.00 ⇒ Value=100). Only Integer values will be accepted!
Example: 100
|
Container "Common_Models_Data_AmountWithZero"
CurrencyCode mandatory, string |
ISO 4217 3-letter currency code (CHF, USD, EUR, ...)
Example: CHF
|
Value mandatory, string |
Amount in minor unit (CHF 1.00 ⇒ Value=100). Only Integer values will be accepted!
Example: 100
|
Container "Common_Models_Data_BillingAddressForm"
AddressSource mandatory, string |
Specifies if and where Saferpay should take the payer's address data from.
Possible values: NONE, SAFERPAY, PREFER_PAYMENTMETHOD.SAFERPAY will result in an address form being shown to the payer in the Saferpay Payment Page. PREFER_PAYMENTMETHOD will retrieve the address data from the means of payment if supported. PREFER_PAYMENTMETHOD will fall back to SAFERPAY if not available with the chosen payment method. For NONE no address form will be displayed and no address data will be retrieved from the means of payment. |
MandatoryFields array of strings |
List of fields which the payer must enter to proceed with the payment.
Possible values: CITY, COMPANY, VATNUMBER, COUNTRY, EMAIL, FIRSTNAME, LASTNAME, PHONE, SALUTATION, STATE, STREET, ZIP.This is only applicable if Saferpay displays the address form. If no mandatory fields are sent, all fields except SALUTATION, COMPANY and PHONE are mandatory. Example: ["FIRSTNAME", "LASTNAME", "PHONE"]
|
Container "Common_Models_Data_DeliveryAddressForm"
AddressSource mandatory, string |
Specifies if and where Saferpay should take the payer's address data from.
Possible values: NONE, SAFERPAY, PREFER_PAYMENTMETHOD.SAFERPAY will result in an address form being shown to the payer in the Saferpay Payment Page. PREFER_PAYMENTMETHOD will retrieve the address data from the means of payment if supported. PREFER_PAYMENTMETHOD will fall back to SAFERPAY if not available with the chosen payment method. For NONE no address form will be displayed and no address data will be retrieved from the means of payment. |
MandatoryFields array of strings |
List of fields which the payer must enter to proceed with the payment.
Possible values: CITY, COMPANY, COUNTRY, EMAIL, FIRSTNAME, LASTNAME, PHONE, SALUTATION, STATE, STREET, ZIP.This is only applicable if Saferpay displays the address form. If no mandatory fields are sent, all fields except SALUTATION, COMPANY and PHONE are mandatory. Example: ["FIRSTNAME", "LASTNAME", "PHONE"]
|
Container "Common_Models_Data_Payer"
AcceptHeader string |
Browser accept header
Example: text/html,application/xhtml\u002Bxml,application/xml
|
BillingAddress object |
Information on the payers billing address
|
ColorDepth string |
Color depth
Possible values: 1bit, 4bits, 8bits, 15bits, 16bits, 24bits, 32bits, 48bits.Example: 32bits
|
DeliveryAddress object |
Information on the payers delivery address
|
Id string |
Payer identifier defined by the merchant / shop. The ID can be numeric, alphabetical and contain any of the following special characters: .:!#$%&'*+-/=?^_`{|}~@.
PayerId[1..256]For GDPR reasons we strongly discourage the use of ids containing any personal data (e.g. names) and instead recommend the usage of a merchant-side generated UUID for your customer. |
IpAddress string |
IPv4 address of the card holder / payer if available. Dotted quad notation.
Example: 111.111.111.111
|
Ipv6Address string |
IPv6 address of the card holder / payer if available.
Example: 2001:0db8:0000:08d3:0000:8a2e:0070:7344
|
JavaEnabled boolean |
Is Java enabled
|
JavaScriptEnabled boolean |
Is JavaScript enabled
|
LanguageCode string |
Language to force Saferpay to display something to the payer in a certain language. Per default, Saferpay will determine the language using the payers browser agent.
Example: Format: ISO 639-1 (two-letter language code), optionally followed by a hyphen and ISO 3166-1 alpha-2 (two-letter country code). The supported language codes are listed below. This list may be extended in the future as more languages become available. We recommend to only use supported language and country codes. If the submitted value has a valid format, but the language is unsupported, then the default language is used. For supported languages, using different country codes than those explicitly listed here may or may not work as expected. Code-List: bg - Bulgarian cs - Czech da - Danish de - German de-CH - Swiss German el - Greek en - English es - Spanish et - Estonian fi - Finnish fr - French hr - Croatian hu - Hungarian is - Icelandic it - Italian ja - Japanese lt - Lithuanian lv - Latvian nl - Dutch nn - Norwegian pl - Polish pt - Portuguese ro - Romanian ru - Russian sk - Slovak sl - Slovenian sv - Swedish tr - Turkish uk - Ukrainian zh - Chinese de
|
ScreenHeight integer |
Screen height
Example: 1080
|
ScreenWidth integer |
Screen width
Example: 1920
|
TimeZoneOffsetMinutes integer |
Time zone offset in minutes
Example: 720
120
|
UserAgent string |
User agent
Example: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:47.0) Gecko/20100101 Firefox/47.0
|
Container "Common_Models_Data_PaymentOptions"
AllowPartialAuthorization boolean |
When set to true, a transaction might be authorized with an amount less than requested authorization amount.
Important:
|
PreAuth boolean |
Indicates the desired transaction type. When set to true the transaction is processed as a pre-authorization otherwise as a final authorization. Please note that not all payment methods support both options and the effective transaction type is determined by Saferpay.
|
Container "Common_Models_Data_RequestAddress"
City string |
The payer's city
Utf8[1..100]Example: Zurich
|
Company string |
The payer's company
Utf8[1..100]Example: ACME Corp.
|
CountryCode string |
The payer's country code
Alphabetic[2..2]ISO 3166-1 alpha-2 country code (Non-standard: XK for Kosovo) Example: CH
|
CountrySubdivisionCode string |
The payer's country subdivision code
Iso3166[1..6]Allows country codes formatted as ISO 3166 or ISO 3166-2. Example: ZH / CH-ZH
|
DateOfBirth string |
The payer's date of birth in ISO 8601 extended date notation
AlphaNumeric[10..10]YYYY-MM-DD Example: 1990-05-31
|
Email string |
The payer's email address
Example: payer@provider.com
|
FirstName string |
The payer's first name
Utf8[1..100]Example: John
|
Gender string |
The payer's gender
Possible values: MALE, FEMALE, DIVERSE, COMPANY.Example: COMPANY
|
LastName string |
The payer's last name
Utf8[1..100]Example: Doe
|
Phone string |
The payer's phone number
Utf8[1..100]Example: +41 12 345 6789
|
Street string |
The payer's street
Utf8[1..100]Example: Bakerstreet 32
|
Street2 string |
The payer's street, second line. Only use this, if you need two lines. It may not be supported by all acquirers.
Utf8[1..100]Example: Stewart House
|
Zip string |
The payer's zip code
Utf8[1..100]Example: 8000
|
Container "Common_Models_Data_RequestBillingAddress"
City string |
The payer's city
Utf8[1..100]Example: Zurich
|
Company string |
The payer's company
Utf8[1..100]Example: ACME Corp.
|
CountryCode string |
The payer's country code
Alphabetic[2..2]ISO 3166-1 alpha-2 country code (Non-standard: XK for Kosovo) Example: CH
|
CountrySubdivisionCode string |
The payer's country subdivision code
Iso3166[1..6]Allows country codes formatted as ISO 3166 or ISO 3166-2. Example: ZH / CH-ZH
|
DateOfBirth string |
The payer's date of birth in ISO 8601 extended date notation
AlphaNumeric[10..10]YYYY-MM-DD Example: 1990-05-31
|
Email string |
The payer's email address
Example: payer@provider.com
|
FirstName string |
The payer's first name
Utf8[1..100]Example: John
|
Gender string |
The payer's gender
Possible values: MALE, FEMALE, DIVERSE, COMPANY.Example: COMPANY
|
LastName string |
The payer's last name
Utf8[1..100]Example: Doe
|
Phone string |
The payer's phone number
Utf8[1..100]Example: +41 12 345 6789
|
Street string |
The payer's street
Utf8[1..100]Example: Bakerstreet 32
|
Street2 string |
The payer's street, second line. Only use this, if you need two lines. It may not be supported by all acquirers.
Utf8[1..100]Example: Stewart House
|
VatNumber string |
The company's vat number
Utf8[1..25] |
Zip string |
The payer's zip code
Utf8[1..100]Example: 8000
|
Container "Common_Models_Data_ResponseAddress"
City string |
The payer's city
Utf8[1..100]Example: Zurich
|
Company string |
The payer's company
Utf8[1..100]Example: ACME Corp.
|
CountryCode string |
The payer's country code
Alphabetic[2..2]ISO 3166-1 alpha-2 country code (Non-standard: XK for Kosovo) Example: CH
|
CountrySubdivisionCode string |
The payer's country subdivision code in ISO 3166-2
AlphaNumeric[1..3]Example: ZH
|
DateOfBirth string |
The payer's date of birth in ISO 8601 extended date notation
AlphaNumeric[10..10]YYYY-MM-DD Example: 1990-05-31
|
Email string |
The payer's email address
Example: payer@provider.com
|
FirstName string |
The payer's first name
Utf8[1..100]Example: John
|
Gender string |
The payer's gender
Possible values: MALE, FEMALE, DIVERSE, COMPANY.Example: COMPANY
|
LastName string |
The payer's last name
Utf8[1..100]Example: Doe
|
Phone string |
The payer's phone number
Utf8[1..100]Example: +41 12 345 6789
|
Street string |
The payer's street
Utf8[1..100]Example: Bakerstreet 32
|
Street2 string |
The payer's street, second line. Only use this, if you need two lines. It may not be supported by all acquirers.
Utf8[1..100]Example: Stewart House
|
Zip string |
The payer's zip code
Utf8[1..100]Example: 8000
|
Container "Common_Models_Data_ResponseBillingAddress"
City string |
The payer's city
Utf8[1..100]Example: Zurich
|
Company string |
The payer's company
Utf8[1..100]Example: ACME Corp.
|
CountryCode string |
The payer's country code
Alphabetic[2..2]ISO 3166-1 alpha-2 country code (Non-standard: XK for Kosovo) Example: CH
|
CountrySubdivisionCode string |
The payer's country subdivision code in ISO 3166-2
AlphaNumeric[1..3]Example: ZH
|
DateOfBirth string |
The payer's date of birth in ISO 8601 extended date notation
AlphaNumeric[10..10]YYYY-MM-DD Example: 1990-05-31
|
Email string |
The payer's email address
Example: payer@provider.com
|
FirstName string |
The payer's first name
Utf8[1..100]Example: John
|
Gender string |
The payer's gender
Possible values: MALE, FEMALE, DIVERSE, COMPANY.Example: COMPANY
|
LastName string |
The payer's last name
Utf8[1..100]Example: Doe
|
Phone string |
The payer's phone number
Utf8[1..100]Example: +41 12 345 6789
|
Street string |
The payer's street
Utf8[1..100]Example: Bakerstreet 32
|
Street2 string |
The payer's street, second line. Only use this, if you need two lines. It may not be supported by all acquirers.
Utf8[1..100]Example: Stewart House
|
VatNumber string |
The company's vat number
Utf8[1..25] |
Zip string |
The payer's zip code
Utf8[1..100]Example: 8000
|
Container "Common_Models_Data_SpgBillingAddressForm"
AddressSource mandatory, string |
Specifies if and where Saferpay should take the payer's address data from.
Possible values: NONE, SAFERPAY, PREFER_PAYMENTMETHOD.SAFERPAY will result in an address form being shown to the payer in the Saferpay Payment Page. PREFER_PAYMENTMETHOD will retrieve the address data from the means of payment if supported. PREFER_PAYMENTMETHOD will fall back to SAFERPAY if not available with the chosen payment method. For NONE no address form will be displayed and no address data will be retrieved from the means of payment. |
MandatoryFields array of strings |
List of fields which the payer must enter to proceed with the payment.
Possible values: CITY, COMPANY, VATNUMBER, COUNTRY, EMAIL, FIRSTNAME, LASTNAME, PHONE, SALUTATION, STATE, STREET, ZIP.This is only applicable if Saferpay displays the address form. If no mandatory fields are sent, all fields except SALUTATION, COMPANY and PHONE are mandatory. Example: ["FIRSTNAME", "LASTNAME", "PHONE"]
|
Container "Common_Models_Data_SpgPayer"
BillingAddress object |
Information on the payers billing address
|
IpAddress string |
IPv4 address of the card holder / payer if available. Dotted quad notation.
Example: 111.111.111.111
|
Ipv6Address string |
IPv6 address of the card holder / payer if available.
Example: 2001:0db8:0000:08d3:0000:8a2e:0070:7344
|
LanguageCode string |
Language to force Saferpay to display something to the payer in a certain language. Per default, Saferpay will determine the language using the payers browser agent.
Example: Format: ISO 639-1 (two-letter language code), optionally followed by a hyphen and ISO 3166-1 alpha-2 (two-letter country code). The supported language codes are listed below. This list may be extended in the future as more languages become available. We recommend to only use supported language and country codes. If the submitted value has a valid format, but the language is unsupported, then the default language is used. For supported languages, using different country codes than those explicitly listed here may or may not work as expected. Code-List: bg - Bulgarian cs - Czech da - Danish de - German de-CH - Swiss German el - Greek en - English es - Spanish et - Estonian fi - Finnish fr - French hr - Croatian hu - Hungarian is - Icelandic it - Italian ja - Japanese lt - Lithuanian lv - Latvian nl - Dutch nn - Norwegian pl - Polish pt - Portuguese ro - Romanian ru - Russian sk - Slovak sl - Slovenian sv - Swedish tr - Turkish uk - Ukrainian zh - Chinese de
|
Container "Common_RequestHeader"
ClientInfo object |
Information about the caller (merchant host)
|
CustomerId mandatory, string |
Saferpay customer id. Part of the Saferpay AccountID, which has the following format: 123123-12345678. The first Value is your CustomerID.
Numeric[1..8]Example: 123123
|
RequestId mandatory, string |
Id generated by merchant system, for debugging purposes. Should be unique for each new request. If a request is retried due to an error, use the same request id. In this case, the RetryIndicator should be increased instead, to indicate a subsequent attempt.
Id[1..50]Example: 33e8af17-35c1-4165-a343-c1c86a320f3b
|
RetryIndicator mandatory, integer |
0 if this specific request is attempted for the first time, >=1 if it is a retry.
Range: inclusive between 0 and 9Example: 0
|
SpecVersion mandatory, string |
Version number of the interface specification. For new implementations, the newest Version should be used.
Possible values: 1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8, 1.9, 1.10, 1.11, 1.12, 1.13, 1.14, 1.15, 1.16, 1.17, 1.18, 1.19, 1.20, 1.21, 1.22, 1.23, 1.24, 1.25, 1.26, 1.27, 1.28, 1.29, 1.30, 1.31, 1.32, 1.33, 1.34, 1.35, 1.36, 1.37, 1.38, 1.39, 1.40, 1.41Example: 1.41
|
Container "Common_ResponseHeader"
RequestId mandatory, string |
RequestId of the original request header
Id[1..50]Example: 33e8af17-35c1-4165-a343-c1c86a320f3b
|
SpecVersion mandatory, string |
Version number of the interface specification.
Possible values: 1.0, 1.1, 1.2, 1.3, 1.4, 1.5, 1.6, 1.7, 1.8, 1.9, 1.10, 1.11, 1.12, 1.13, 1.14, 1.15, 1.16, 1.17, 1.18, 1.19, 1.20, 1.21, 1.22, 1.23, 1.24, 1.25, 1.26, 1.27, 1.28, 1.29, 1.30, 1.31, 1.32, 1.33, 1.34, 1.35, 1.36, 1.37, 1.38, 1.39, 1.40, 1.41Example: 1.41
|
Container "Payment_Models_A2ARefund"
AccountHolderName mandatory, string |
The account holder name will be used if not already present in the authorization.
Utf8[1..50]If no account holder name is present in the authorization and none provided in the refund request, the refund cannot be processed. Example: John Doe
|
Container "Payment_Models_AlternativePayment_BancontactPaymentMethodOptions"
AppCompletionRedirectUrl URI |
This URL is called by the bancontact payment app once the payment is authorized successfully.
The maximum allowed length for this URL is 256 characters. |
AppDefaultRedirectUrl URI |
This URL is called by the bancontact payment app when the payer cancels the payment.
The maximum allowed length for this URL is 256 characters. |
Container "Payment_Models_AlternativePayment_PaymentMethodOptions"
Bancontact object |
bancontact-specific options for this payment
|
Container "Payment_Models_CaptureReference"
CaptureId string |
Id of the referenced capture.
Id[1..64]Example: jCUC8IAQ1OCptA5I8jpzAMxC5nWA_c
|
OrderId string |
Unambiguous OrderId of the referenced transaction.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
OrderPartId string |
OrderPartId of the referenced capture if a partial capture should be referenced and the CaptureId/TransactionId of the partial capture is not available.
Id[1..80]Example: kh9ngajrfe6wfu3d0c
|
TransactionId string |
Id of the referenced transaction. This should only be used if you don't have the CaptureId of the referenced Capture (probably, because it was performed with an older SpecVersion)
AlphaNumeric[1..64]Example: 723n4MAjMdhjSAhAKEUdA8jtl9jb
|
Container "Payment_Models_Data_Alias"
Id mandatory, string |
Id / name of the alias. This value is case-insensitive.
Id[1..40]Example: alias35nfd9mkzfw0x57iwx
|
VerificationCode string |
Verification code (CVV, CVC) if applicable (if the alias referenced is a card).
Numeric[3..4]Example: 123
|
Container "Payment_Models_Data_AliasAuthenticationResult"
Message mandatory, string |
More details, if available. Contents may change at any time, so don’t parse it.
Example: Card holder authentication with 3DSv2 successful.
|
Result mandatory, string |
The result of the card holder authentication.
Possible values: OK, NOT_SUPPORTED. |
Xid string |
Transaction Id, given by the MPI. References the 3D Secure version 2 process.
Example: 1ef5b3db-3b97-47df-8272-320d0bd18ab5
|
Container "Payment_Models_Data_AliasAuthenticationResultBase"
Message mandatory, string |
More details, if available. Contents may change at any time, so don’t parse it.
Example: Card holder authentication with 3DSv2 successful.
|
Result mandatory, string |
The result of the card holder authentication.
Possible values: OK, NOT_SUPPORTED. |
Container "Payment_Models_Data_AliasDirectInsertCheck"
TerminalId mandatory, string |
Saferpay Terminal-Id to be used for checking.
Numeric[8..8]Example: 12341234
|
Type mandatory, string |
Type of check to perform.
Possible values: ONLINE.Example: ONLINE
|
Container "Payment_Models_Data_AliasInfo"
Id mandatory, string |
Id / name of the alias
Example: alias35nfd9mkzfw0x57iwx
|
Lifetime mandatory, integer |
Number of days this card is stored within Saferpay. Minimum 1 day, maximum 1600 days.
Example: 1000
|
Container "Payment_Models_Data_AliasInsertCheck"
TerminalId mandatory, string |
Saferpay Terminal-Id to be used for checking.
Numeric[8..8]Example: 12341234
|
Type mandatory, string |
Type of check to perform (subject to availability for the brand/acquirer).
Possible values: ONLINE, ONLINE_STRONG.ONLINE performs an online account check (one transaction point will be billed for such a check) ONLINE_STRONG performs a strong customer authentication (SCA) which involves a 3DS v2 authentication and an online account check (for which one transaction point will be billed) Example: ONLINE
|
Container "Payment_Models_Data_AliasInsertCheckResult"
Authentication object |
More details about the card holder authentication.
|
IssuerReference object |
|
Message mandatory, string |
More details, if available. Contents may change at any time, so don’t parse it.
Example: Online card check was successful.
|
Result mandatory, string |
The result of the card check.
Possible values: OK, OK_AUTHENTICATED, NOT_PERFORMED. |
Container "Payment_Models_Data_AliasInsertDirectPaymentMeans"
Alias object |
Alias data if payment means was registered with Secure Card Data before.
|
ApplePay object |
Payment Data from ApplePay
|
BankAccount object |
Bank account data for direct debit transaction
|
Card object |
Card data
|
GooglePay object |
Payment Data from GooglePay
|
SchemeToken object |
Surrogate values that replace the primary account number (PAN) according to the EMV Payment Tokenization Specification.
Note: LiabilityShift is only possible with Transaction Initialize, AuthorizeDirect and RefundDirect. Note: Scheme tokens MDES and VTS are only available for Worldline Acquiring. |
Container "Payment_Models_Data_AliasNotification"
NotifyUrl string |
the url that should be called to notify when the process is done
|
Container "Payment_Models_Data_AliasPaymentMeansInfo"
BankAccount object |
Bank account data for direct debit transactions.
|
Brand mandatory, object |
Brand information
|
Card object |
Card data
|
DisplayText mandatory, string |
Means of payment formatted / masked for display purposes
Example: DisplayText
|
Twint object |
Twint data
|
Wallet string |
Name of Wallet, if the transaction was done by a wallet
Example: APPLEPAY
|
Container "Payment_Models_Data_AlternativePaymentNotification"
MerchantEmails array of strings |
Email addresses to which a confirmation email will be sent to the merchants after successful authorizations.
Example: A maximum of 10 email addresses is allowed. ["merchant1@saferpay.com", "merchant2@saferpay.com"]
|
PayerEmail string |
Email address to which a confirmation email will be sent to the payer after successful authorizations.
Example: payer@saferpay.com
|
StateNotificationUrl mandatory, URI |
Url to which Saferpay will send the asynchronous confirmation for the transaction. Supported schemes are http and https. You also have to make sure to support the GET-method.
Example: https://merchanthost/notify
|
Container "Payment_Models_Data_AlternativePaymentProcessingData"
Bancontact object |
Bancontact specific data for processing payment
|
Container "Payment_Models_Data_ApplePay"
PaymentToken mandatory, string |
Base64 encoded ApplePayPaymentToken Json according to Apple's Payment Token Format Reference
Base64 encoded string |
Container "Payment_Models_Data_AuthorizeDirectPaymentMeans"
Alias object |
Alias data if payment means was registered with Secure Card Data before.
|
ApplePay object |
Payment Data from Apple Pay Wallet
|
BankAccount object |
Bank account data for direct debit transaction
|
Card object |
Card data
|
GooglePay object |
Payment Data from GooglePay
|
SaferpayFields object |
Payment means data collected with SaferpayFields.
|
SchemeToken object |
Surrogate values that replace the primary account number (PAN) according to the EMV Payment Tokenization Specification.
Note: LiabilityShift is only possible with Transaction Initialize, AuthorizeDirect and RefundDirect. Note: Scheme tokens MDES and VTS are only available for Worldline Acquiring. |
Container "Payment_Models_Data_BancontactProcessingData"
IntentUrl string |
Url to be used for payment on the same device (web-to-app or app-to-app switch)
|
QrCodeData string |
Data which should be integrated into a QR code. In order to make the scanning as easy as possible,
the recommended format of QR code encoding is version 3, with lower error rate correction level in character mode, resulting with a 29 x 29 pixels image maximum. |
Container "Payment_Models_Data_BankAccount"
BankName string |
Name of the Bank.
|
BIC string |
Bank Identifier Code without spaces.
AlphaNumeric[8..11]Example: INGDDEFFXXX
|
HolderName string |
Name of the account holder.
Iso885915[1..50]Example: John Doe
|
IBAN mandatory, string |
International Bank Account Number in electronical format (without spaces).
AlphaNumeric[1..50]Example: DE12500105170648489890
|
Container "Payment_Models_Data_BankAccountInfo"
BankName string |
Name of the Bank.
|
BIC string |
Bank Identifier Code without spaces.
AlphaNumeric[8..11]Example: INGDDEFFXXX
|
CountryCode string |
ISO 2-letter country code of the IBAN origin (if available)
Example: CH
|
HolderName string |
Name of the account holder.
Iso885915[1..50]Example: John Doe
|
IBAN mandatory, string |
International Bank Account Number in electronical format (without spaces).
AlphaNumeric[1..50]Example: DE12500105170648489890
|
Container "Payment_Models_Data_BasicPayment"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description string |
A human readable description provided by the merchant that can be displayed in web sites.
Utf8[1..1000]Example: Description of payment
|
OrderId recommended, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
PayerNote string |
Text which will be printed on payer's debit note. Supported by SIX Acquiring. No guarantee that it will show up on the payer's debit note, because his bank has to support it too.
Utf8[1..50]Please note that maximum allowed characters are rarely supported. It's usually around 10-12. Example: Payernote
|
Container "Payment_Models_Data_BillpayCapture"
DelayInDays integer |
Number of days to delay the due date of the invoice / direct debit (see billpay for specifics)
Example: 10
|
Container "Payment_Models_Data_Brand"
Name mandatory, string |
Name of the Brand (Visa, Mastercard, an so on – might change over time, only use for display, never for parsing). Only use it for display, never for parsing and/or mapping! Use PaymentMethod instead.
Example: VISA
|
PaymentMethod string |
alphanumeric id of the payment method / brand
Possible values: ACCOUNTTOACCOUNT, ALIPAY, AMEX, BANCONTACT, BONUS, DINERS, CARD, DIRECTDEBIT, EPRZELEWY, EPS, GIROPAY, IDEAL, INVOICE, JCB, KLARNA, MAESTRO, MASTERCARD, MYONE, PAYCONIQ, PAYDIREKT, PAYPAL, POSTCARD, POSTFINANCE, POSTFINANCEPAY, SOFORT, TWINT, UNIONPAY, VISA, WECHATPAY, WLCRYPTOPAYMENTS. |
Container "Payment_Models_Data_Card"
ExpMonth mandatory, integer |
Month of expiration (eg 9 for September)
Range: inclusive between 1 and 12Example: 9
|
ExpYear mandatory, integer |
Year of expiration
Range: inclusive between 2000 and 9999Example: 2015
|
HolderName string |
Name of the card holder
Utf8[1..50]Example: John Doe
|
Number mandatory, string |
Card number without separators
Example: 1234123412341234
|
VerificationCode string |
Verification code (CVV, CVC) if applicable
Numeric[3..4]Example: 123
|
Container "Payment_Models_Data_CardForm"
HolderName string |
This parameter lets you customize the holder name field on the card entry form. Per default, a holder name field is not shown.
Possible values: NONE, MANDATORY.Example: MANDATORY
|
Container "Payment_Models_Data_CardFormInTransactionInitialize"
HolderName string |
This parameter lets you customize the holder name field on the card entry form. Per default, a holder name field is not shown.
Possible values: NONE, MANDATORY.Example: MANDATORY
|
VerificationCode string |
This parameter can be used to display an entry form to request Verification Code (CVV, CVC) in case that an alias is used as PaymentMeans. Note that not all brands support Verification Code.
Possible values: NONE, MANDATORY.Example: MANDATORY
|
Container "Payment_Models_Data_CardInfo"
CountryCode string |
ISO 2-letter country code of the card origin (if available)
Example: CH
|
ExpMonth mandatory, integer |
Month of expiration (eg 9 for September)
Example: 9
|
ExpYear mandatory, integer |
Year of expiration
Example: 2015
|
HashValue string |
The HashValue, if the hash generation is configured for the customer.
|
HolderName string |
Name of the card holder (if known)
Example: John Doe
|
HolderSegment string |
The Segment of card holder. Only returned for Alias/AssertInsert, Alias/InsertDirect and Alias/Update calls if available.
Possible values: UNSPECIFIED, CONSUMER, CORPORATE, CORPORATE_AND_CONSUMER.Example: CORPORATE
|
MaskedNumber mandatory, string |
Masked card number
Example: 912345xxxxxx1234
|
Container "Payment_Models_Data_ClientInfo"
OsInfo string |
Information on the operating system
Iso885915[1..100]Example: Windows Server 2013
|
ShopInfo string |
Name and version of the shop software
Iso885915[1..100]Example: My Shop
|
Container "Payment_Models_Data_CustomPlan"
AnnualPercentageRate string |
Annual percentage rate in hundreth of a percent. e.g. value 125 means 1.25%.
Valid values are 0-99999 |
InstallmentFee object |
Installment Fee
|
InterestRate string |
Interest rate in hundredth of a percent. e.g. value 125 means 1.25%.
Valid values are 0-99999 |
MaximumNumberOfInstallments mandatory, integer |
Minimum Number of Installments. Valid values are 2–99 and
MinimumNumberOfInstallments must be smaller or equal MaximumNumberOfInstallments. |
MinimumNumberOfInstallments mandatory, integer |
Maximum Number of Installments. Valid values are 2–99 and
MaximumNumberOfInstallments must be bigger or equal MinimumNumberOfInstallments. |
TotalAmountDue object |
Total Amount Due
|
Container "Payment_Models_Data_DccInfo"
ExchangeRate mandatory, string |
The used exchange rate including markup
Example: The decimal separator used is '.' regardless of location 1.2345
|
Markup mandatory, string |
DCC markup fee
Example: 3%
|
PayerAmount mandatory, object |
Amount in payer’s currency
|
Container "Payment_Models_Data_DirectDebitInfo"
CreditorId mandatory, string |
Creditor id, required for german direct debit payments.
|
MandateId mandatory, string |
The unique Mandate reference, required for german direct debit payments.
|
Container "Payment_Models_Data_ForeignRetailerMerchantInformation"
City string |
City of merchant's location
Utf8[1..13] |
CountryCode string |
country code of merchant's location
Alphabetic[2..2]ISO 3166-1 alpha-2 country code if not submitted the container will be ignored Example: CH
|
Name string |
Name of merchant
Utf8[1..14] |
Street string |
Street of merchant's location
Utf8[1..48] |
Zip string |
Postal code of merchant's location
Utf8[1..10] |
Container "Payment_Models_Data_GooglePay"
PaymentToken mandatory, string |
GooglePay Payment Token
|
Container "Payment_Models_Data_HostedFormStyling"
ContentSecurityEnabled boolean |
When enabled, then ContentSecurity/SAQ-A is requested, which leads to the CSS being loaded from the saferpay server.
|
CssUrl string |
Custom styling resource (url) which will be referenced in web pages displayed by Saferpay to apply your custom styling.
Max length: 2000This file must be hosted on a SSL/TLS secured web server (the url must start with https://). If a custom CSS is provided, any design related settings set in the payment page config (PPConfig) will be ignored and the default design will be used. Example: https://merchanthost/merchant.css
|
Theme string |
This parameter let you customize the appearance of the displayed payment pages. Per default a lightweight responsive styling will be applied.
Possible values: DEFAULT, SIX, NONE.If you don't want any styling use 'NONE'. Example: DEFAULT
|
Container "Payment_Models_Data_IdealOptions"
IssuerId mandatory, string |
Preselect the iDEAL issuer. If specified together with PaymentMethods preselection of iDEAL,
the user is redirected directly to the issuer bank. If the IssuerId is set, it is mandatory to use iDEAL in PaymentMethods. |
Container "Payment_Models_Data_InsertAliasSupportingPaymentMeans"
Card object |
Card data
|
SaferpayFields object |
Payment means data collected with SaferpayFields.
|
Container "Payment_Models_Data_InstallmentOptions"
Initial mandatory, boolean |
If set to true, the authorization may later be referenced for installment followup transactions.
|
Container "Payment_Models_Data_InstallmentPlan"
AnnualPercentageRate string |
Annual percentage rate in hundredth of a percent. e.g. value 125 means 1.25%.
Valid values are 0-99999 |
FirstInstallmentAmount object |
First Installment Amount
|
InstallmentFee object |
Installment Fee
|
InterestRate string |
Interest rate in hundredth of a percent. e.g. value 125 means 1.25%.
Valid values are 0-99999 |
NumberOfInstallments mandatory, integer |
Number of Installments. Valid values are 2–99.
Range: inclusive between 2 and 99 |
SubsequentInstallmentAmount object |
Subsequent Installment Amount
|
TotalAmountDue object |
Total Amount Due
|
Container "Payment_Models_Data_InvoiceInfo"
DueDate date |
The date by which the invoice needs to be settled
|
Payee object |
Information about the payee, eg billpay, who is responsible for collecting the bill
|
ReasonForTransfer string |
The reason for transfer to be stated when paying the invoice (transfer of funds)
|
Container "Payment_Models_Data_IssuerReference"
SettlementDate string |
SettlementDate with the format MMDD
String length: inclusive between 4 and 4Example: 0122
|
TransactionStamp mandatory, string |
TransactionStamp
Max length: 50Example: 9406957728464714731817
|
Container "Payment_Models_Data_IssuerReferenceInfo"
SettlementDate string |
SCA transaction settlement date, created by the card issuer. For MasterCard schemes only.
|
TransactionStamp string |
SCA transaction stamp, created by the card issuer
|
Container "Payment_Models_Data_KlarnaAttachment"
Body mandatory, string |
Utf8[1..100000] |
ContentType mandatory, string |
Utf8[1..1000] |
Container "Payment_Models_Data_KlarnaOptions"
Attachment mandatory, object |
Klarna extra merchant data (EMD).
Check Klarna's EMD documentation for further details. |
Container "Payment_Models_Data_LiabilityInfo"
InPsd2Scope string |
Determines if the transaction is in the PSD2 Scope (Payment Service Directive 2 of the European Union)
Possible values: YES, NO, UNKNOWN. |
LiabilityShift mandatory, boolean |
Is liability shifted for this transaction
|
LiableEntity mandatory, string |
Indicates who takes the liability for the transaction
Possible values: MERCHANT, THREEDS. |
ThreeDs object |
Details about ThreeDs if applicable
|
Container "Payment_Models_Data_MarketplaceCapture"
Fee object |
The marketplace fee that will be charged from the marketplace to the submerchant.
The properties Fee and FeeRefund cannot be used simultaneously. |
FeeRefund object |
The fee amount that will be refunded from the marketplace to the submerchant.
The properties Fee and FeeRefund cannot be used simultaneously. |
ForeignRetailer object |
Merchant information required for foreign retailers Mandatory for foreign retailers of an international marketplace |
SubmerchantId mandatory, string |
The id of the marketplace submerchant on whose behalf a multipart capture or refund capture is being made.
Id[1..15] |
Container "Payment_Models_Data_MastercardIssuerInstallmentsOptions"
CustomPlan object |
An installment plan with customizable numbers of installments
If CustomPlan is present, InstallmentPlans must not be present An installment plan with customizable numbers of installments |
InstallmentPlans array of objects |
A maximum number of 12 fixed installment plans
If InstallmentPlans is present, CustomPlan must not be present |
ReceiptFreeText string |
Receipt Free Text
|
Container "Payment_Models_Data_MastercardIssuerInstallmentsSelection"
ChosenPlan object |
Installment Payment Data, if applicable
A single, fixed installment plan |
Container "Payment_Models_Data_Notification"
FailNotifyUrl recommended, string |
Url to which Saferpay will send the asynchronous failure notification for the transaction. Supported schemes are http and https. You also have to make sure to support the GET-method.
Max length: 2000Example: https://merchanthost/notify/123
|
MerchantEmails array of strings |
Email addresses to which a confirmation email will be sent to the merchants after successful authorizations.
Example: A maximum of 10 email addresses is allowed. ["merchant1@saferpay.com", "merchant2@saferpay.com"]
|
PayerDccReceiptEmail string |
Email address to which a confirmation email will be sent to the payer after successful authorizations processed with DCC.
Example: This option can only be used when the field PayerEmail is not set. payer@saferpay.com
|
PayerEmail string |
Email address to which a confirmation email will be sent to the payer after successful authorizations.
Example: payer@saferpay.com
|
SuccessNotifyUrl recommended, string |
Url to which Saferpay will send the asynchronous success notification for the transaction. Supported schemes are http and https. You also have to make sure to support the GET-method.
Max length: 2000Example: https://merchanthost/notify/123
|
Container "Payment_Models_Data_Order"
Items mandatory, array of objects |
Order items
|
Container "Payment_Models_Data_OrderItem"
CategoryName string |
Category name
Utf8[1..100]Example: knives
|
Description string |
Product description
Utf8[1..200]Example: The well known swiss army knife
|
DiscountAmount string |
Discount amount including tax
Example: 92
|
Id string |
Product id
Utf8[1..100]Example: C123192
|
ImageUrl string |
URL to an image showing the product
Max length: 2000Example: https://merchanthost/product/1/image
|
IsPreOrder boolean |
Flag, which indicates that the order item is a pre order. Per default, it is not a pre order.
Example: true
|
Name string |
Product name
Utf8[1..200]Example: red swiss army knife
|
ProductUrl string |
URL to the product page
Max length: 2000Example: https://merchanthost/product/1
|
Quantity integer |
The quantity of the order item
Example: 2
|
TaxAmount string |
Total tax amount of the order item. This tax needs to be included in the UnitPrice and must take the Quantity of the order item into account.
Example: 480
|
TaxRate string |
Tax rate of the item price in hundredth of a percent. e.g. value 1900 means 19.00%
Range: inclusive between 0 and 99999Valid values are 0-99999 Example: 1900
|
Type string |
Order item type
Possible values: DIGITAL, PHYSICAL, SERVICE, GIFTCARD, DISCOUNT, SHIPPINGFEE, SALESTAX, SURCHARGE. |
UnitPrice string |
Price per single item in minor unit (CHF 15.50 ⇒ Value=1550). Only Integer values will be accepted!
Example: 1550
|
VariantId string |
Product variant id
Utf8[1..100]Example: C123192-red
|
Container "Payment_Models_Data_OriginalCreditTransfer"
Recipient object |
Address of the Recipient.
|
Container "Payment_Models_Data_P2PEnabledPayment"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description string |
A human readable description provided by the merchant that can be displayed in web sites.
Utf8[1..1000]Example: Description of payment
|
Installment object |
Installment options – cannot be combined with Recurring.
|
MandateId string |
Mandate reference of the payment. Needed for German direct debits (ELV) only. The value has to be unique.
Id[1..35]Example: MandateId
|
Options object |
Specific payment options
|
OrderId recommended, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
PayerNote string |
Text which will be printed on payer's debit note. Supported by SIX Acquiring. No guarantee that it will show up on the payer's debit note, because his bank has to support it too.
Utf8[1..50]Please note that maximum allowed characters are rarely supported. It's usually around 10-12. Example: Payernote
|
Recurring object |
Recurring options – cannot be combined with Installment.
|
Container "Payment_Models_Data_P2PEnabledPaymentMeansInfo"
BankAccount object |
Bank account data for direct debit transactions.
|
Brand mandatory, object |
Brand information
|
Card object |
Card data
|
DisplayText mandatory, string |
Means of payment formatted / masked for display purposes
Example: DisplayText
|
PayPal object |
PayPal data
|
Wallet string |
Name of Wallet, if the transaction was done by a wallet
Example: APPLEPAY
|
Container "Payment_Models_Data_PayerInfo"
BillingAddress object |
|
DeliveryAddress object |
|
Id string |
Payer identifier defined by the merchant / shop. The ID can be numeric, alphabetical and contain any of the following special characters: .:!#$%&'*+-/=?^_`{|}~@.
PayerId[1..256] |
IpAddress string |
IPv4 address of the card holder / payer if available. Dotted quad notation.
Example: 111.111.111.111
|
IpLocation string |
The location the IpAddress, if available. This might be a valid country code or a special value for 'non-country' locations (anonymous proxy, satellite phone, ...).
Example: NZ
|
Container "Payment_Models_Data_PayerProfile"
Company string |
The payer's company
Utf8[1..100] |
CreationDate date |
Date and Time (ISO 8601) when user account was created
Example: 2018-05-25T18:12:43Z
2018-05-25T19:12:43+01:00
|
DateOfBirth string |
The payer's date of birth (ISO 8601)
AlphaNumeric[10..10]YYYY-MM-DD Example: 1990-05-31
|
Email string |
The payer's email address
Example: payer@provider.com
|
FirstName string |
The payer's first name
Utf8[1..100] |
Gender string |
The payer's gender
Possible values: MALE, FEMALE, DIVERSE, COMPANY.Example: COMPANY
|
HasAccount boolean |
Does the payer have an account in the shop?
|
HasPassword boolean |
Does the payer have a password?
|
LastLoginDate date |
The payer's last login (ISO 8601)
Example: 2018-05-25T18:12:43Z
2018-05-25T19:12:43+01:00
|
LastName string |
The payer's last name
Utf8[1..100] |
PasswordForgotten boolean |
Was the password reset by the payer using the "forgot my password" feature in the current session?
|
PasswordLastChangeDate date |
Date and Time (ISO 8601) when the account password was changed last time
Example: 2018-05-25T18:12:43Z
2018-05-25T19:12:43+01:00
|
Phone object |
The payer's phone numbers
|
SecondaryEmail string |
The payer's secondary email address
Example: payer_secondary@provider.com
|
Container "Payment_Models_Data_Payment"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description string |
A human readable description provided by the merchant that can be displayed in web sites.
Utf8[1..1000]Example: Description of payment
|
Installment object |
Installment options – cannot be combined with Recurring.
|
MandateId string |
Mandate reference of the payment. Needed for German direct debits (ELV) only. The value has to be unique.
Id[1..35]Example: MandateId
|
Options object |
Specific payment options
|
OrderId recommended, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
PayerNote string |
Text which will be printed on payer's debit note. Supported by SIX Acquiring. No guarantee that it will show up on the payer's debit note, because his bank has to support it too.
Utf8[1..50]Please note that maximum allowed characters are rarely supported. It's usually around 10-12. Example: Payernote
|
Recurring object |
Recurring options – cannot be combined with Installment.
|
Container "Payment_Models_Data_PaymentMeans"
Alias object |
Alias data if payment means was registered with Secure Card Data before.
|
ApplePay object |
Payment Data from ApplePay
|
BankAccount object |
Bank account data for direct debit transaction
|
Card object |
Card data
|
GooglePay object |
Payment Data from GooglePay
|
SaferpayFields object |
Payment means data collected with SaferpayFields.
|
SchemeToken object |
Surrogate values that replace the primary account number (PAN) according to the EMV Payment Tokenization Specification.
Note: LiabilityShift is only possible with Transaction Initialize, AuthorizeDirect and RefundDirect. Note: Scheme tokens MDES and VTS are only available for Worldline Acquiring. |
Container "Payment_Models_Data_PaymentMeansInfo"
BankAccount object |
Bank account data for direct debit transactions.
|
Brand mandatory, object |
Brand information
|
Card object |
Card data
|
DisplayText mandatory, string |
Means of payment formatted / masked for display purposes
Example: DisplayText
|
PayPal object |
PayPal data
|
Wallet string |
Name of Wallet, if the transaction was done by a wallet
Example: APPLEPAY
|
Container "Payment_Models_Data_PaymentPagePayment"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description mandatory, string |
A human readable description provided by the merchant that will be displayed in Payment Page.
Utf8[1..1000]Example: Description of payment
|
Installment object |
Installment options – cannot be combined with Recurring.
|
MandateId string |
Mandate reference of the payment. Needed for German direct debits (ELV) only. The value has to be unique.
Id[1..35]Example: MandateId
|
Options object |
Specific payment options
|
OrderId recommended, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
PayerNote string |
Text which will be printed on payer's debit note. Supported by SIX Acquiring. No guarantee that it will show up on the payer's debit note, because his bank has to support it too.
Utf8[1..50]Please note that maximum allowed characters are rarely supported. It's usually around 10-12. Example: Payernote
|
Recurring object |
Recurring options – cannot be combined with Installment.
|
Container "Payment_Models_Data_PaymentTransaction"
AcquirerName string |
Name of the acquirer
Example: AcquirerName
|
AcquirerReference string |
Reference id of the acquirer (if available)
Example: AcquirerReference
|
Amount mandatory, object |
Amount (currency, value, etc.) that has been authorized.
|
ApprovalCode string |
Approval id of the acquirer (if available)
Example: 109510
|
CaptureId string |
Unique Saferpay capture id.
Id[1..64]Available if the transaction was already captured (Status: CAPTURED). Must be stored for later reference (eg refund). Example: ECthWpbv1SI6SAIdU2p6AIC1bppA
|
Date mandatory, date |
Date / time of the authorization
Example: 2011-09-23T14:57:23.023+02.00
|
DirectDebit object |
Direct Debit information, if applicable
Example: AcquirerReference
|
Id mandatory, string |
Unique Saferpay transaction id. Used to reference the transaction in any further step.
Example: K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Invoice object |
Invoice information, if applicable
|
IssuerReference object |
Issuer reference information, if applicable
|
OrderId string |
OrderId given with request
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
SixTransactionReference mandatory, string |
Unique SIX transaction reference.
Example: 0:0:3:K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Status mandatory, string |
Current status of the transaction. One of 'AUTHORIZED','CANCELED', 'CAPTURED' or 'PENDING'
Possible values: AUTHORIZED, CANCELED, CAPTURED, PENDING.Example: AUTHORIZED
|
Type mandatory, string |
Type of transaction. One of 'PAYMENT'
Possible values: PAYMENT.Example: PAYMENT
|
Container "Payment_Models_Data_PayPalInfo"
Email mandatory, string |
The email address used in PayPal
|
PayerId mandatory, string |
PayerId from PayPal
Example: 5b9aefc5-9b48-4a95-ae47-cda20420d68e
|
SellerProtectionStatus mandatory, string |
Seller protection status from PayPal.
Possible values: ELIGIBLE, PARTIALLY_ELIGIBLE, NOT_ELIGIBLE.Example: ELIGIBLE
|
Container "Payment_Models_Data_PendingNotification"
MerchantEmails array of strings |
Email addresses to which a confirmation email will be sent when the transaction is completed.
Example: A maximum of 10 email addresses is allowed. ["merchant1@saferpay.com", "merchant2@saferpay.com"]
|
NotifyUrl recommended, string |
Url which is called by Saferpay if an action could not be completed synchronously and was reported with a ‘pending’ state (eg CAPTURE_PENDING or REFUND_PENDING). Up until now, this is only applicable for Paydirekt transactions or WL Crypto Payments refunds.
Example: https://merchanthost/pendingnotify
|
Container "Payment_Models_Data_Phone"
Main string |
The payer's main phone number
Utf8[1..100]Example: +41 12 345 6789
|
Mobile string |
The payer's mobile number
Utf8[1..100]Example: +41 79 345 6789
|
Work string |
The payer's work phone number
Utf8[1..100]Example: +41 12 345 6789
|
Container "Payment_Models_Data_RecurringOptions"
Initial mandatory, boolean |
If set to true, the authorization may later be referenced for recurring followup transactions.
|
Container "Payment_Models_Data_Redirect"
PaymentMeansRequired mandatory, boolean |
If 'true', the given URL must either be used as the target of a form (POST) containing card data entered by the card holder or to redirect the browser to (GET). If ‘false’, a GET redirect without additional data must be performed.
|
RedirectUrl mandatory, string |
Redirect-URL. Used to either redirect the payer or let him enter his means of payment.
Example: https://www.saferpay.com/VT2/api/...
|
Container "Payment_Models_Data_RedirectNotifyUrls"
Fail mandatory, string |
Notification url called when the payer redirect steps have failed. The transaction cannot be authorized.
Max length: 2000Example: https://merchanthost/fail/123
|
Success mandatory, string |
Notification url called when the payer has completed the redirect steps successfully and the transaction is ready to be authorized.
Max length: 2000Example: https://merchanthost/success/123
|
Container "Payment_Models_Data_Refund"
Amount mandatory, object |
Amount data
|
Description string |
Description provided by merchant
Utf8[1..1000]Example: Description
|
OrderId recommended, string |
Reference defined by the merchant.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
RestrictRefundAmountToCapturedAmount boolean |
If set to true, the refund will be rejected if the sum of refunds exceeds the captured amount.
All authorized refunds are included in the calculation even if they have not been captured yet. Cancelled refunds are not included. By default, this check is disabled. |
Container "Payment_Models_Data_RefundDirect"
Amount mandatory, object |
Amount data
|
Description string |
Description provided by merchant
Utf8[1..1000]Example: Description
|
OrderId recommended, string |
Reference defined by the merchant.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
Container "Payment_Models_Data_RefundDirectSupportingPaymentMeans"
Alias object |
Alias data if payment means was registered with Secure Card Data before.
|
Card object |
Card data
|
SaferpayFields object |
Payment means data collected with SaferpayFields.
|
SchemeToken object |
Surrogate values that replace the primary account number (PAN) according to the EMV Payment Tokenization Specification.
Note: LiabilityShift is only possible with Transaction Initialize, AuthorizeDirect and RefundDirect. Note: Scheme tokens MDES and VTS are only available for Worldline Acquiring. |
Container "Payment_Models_Data_RefundTransaction"
AcquirerName string |
Name of the acquirer
Example: AcquirerName
|
AcquirerReference string |
Reference id of the acquirer (if available)
Example: AcquirerReference
|
Amount mandatory, object |
Amount (currency, value, etc.) that has been authorized.
|
ApprovalCode string |
Approval id of the acquirer (if available)
Example: 109510
|
CaptureId string |
Unique Saferpay capture id.
Id[1..64]Available if the transaction was already captured (Status: CAPTURED). Must be stored for later reference (eg refund). Example: ECthWpbv1SI6SAIdU2p6AIC1bppA
|
Date mandatory, date |
Date / time of the authorization
Example: 2011-09-23T14:57:23.023+02.00
|
DirectDebit object |
Direct Debit information, if applicable
Example: AcquirerReference
|
Id mandatory, string |
Unique Saferpay transaction id. Used to reference the transaction in any further step.
Example: K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Invoice object |
Invoice information, if applicable
|
IssuerReference object |
Issuer reference information, if applicable
|
OrderId string |
OrderId given with request
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
SixTransactionReference mandatory, string |
Unique SIX transaction reference.
Example: 0:0:3:K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Status mandatory, string |
Current status of the transaction. One of 'AUTHORIZED','CANCELED', 'CAPTURED' or 'PENDING'
Possible values: AUTHORIZED, CANCELED, CAPTURED, PENDING.Example: AUTHORIZED
|
Type mandatory, string |
Type of transaction. One of 'REFUND'
Possible values: REFUND.Example: REFUND
|
Container "Payment_Models_Data_RegisterAlias"
Id string |
Alias id to be used for registration if not generated by Saferpay. Mandatory if IdGenerator is 'MANUAL'. This value is case-insensitive.
Id[1..40]Example: alias35nfd9mkzfw0x57iwx
|
IdGenerator mandatory, string |
Id generator to be used by Saferpay.
Possible values: MANUAL, RANDOM, RANDOM_UNIQUE.Example: MANUAL
|
Lifetime integer |
Number of days this card is to be stored within Saferpay. If not filled, the default lifetime (1096 days) will be used.
Range: inclusive between 1 and 1600Example: 1000
|
Container "Payment_Models_Data_RegistrationErrorInfo"
ErrorMessage string |
Description of the error.
Example: ErrorMessage
|
ErrorName string |
Name / id of the error.
Example: ErrorName
|
Container "Payment_Models_Data_RegistrationResult"
Alias object |
If Success is 'true', information about the alias
|
AuthenticationResult object |
contains information whether the alias is saved with the strong authentication details or not.
|
Error object |
If Success is 'false', information on why the registration failed
|
Success mandatory, boolean |
Result of registration
|
Container "Payment_Models_Data_ReturnUrl"
Url mandatory, string |
Return url for successful, failed or aborted transaction
Max length: 2000Example: https://merchanthost/return
|
Container "Payment_Models_Data_RiskFactors"
DeliveryType string |
Delivery method
Possible values: EMAIL, SHOP, HOMEDELIVERY, PICKUP, HQ. |
DeviceFingerprintTransactionId string |
The customer's Session ID (mastered by DFP Device Fingerprinting), or the event ID if the session is not available
Max length: 1024 |
IsB2B boolean |
Is the transaction B2B?
|
PayerProfile object |
Information on the payer executing the transaction, generally referring to his/her customer profile in the shop (if any).
|
Container "Payment_Models_Data_SaferpayFields"
Token mandatory, string |
Saferpay Fields token
Id[1..40] |
Container "Payment_Models_Data_SchemeToken"
AuthValue string |
TAVV Cryptogram
|
Eci string |
Saferpay E-Commerce Indicator. This indicator is just informational.
Example: 07
|
ExpMonth mandatory, integer |
Expiry Month of the token.
Range: inclusive between 1 and 12Example: 10
|
ExpYear mandatory, integer |
Expiry Year of the token.
Example: 2023
|
Number mandatory, string |
Token number
|
TokenType mandatory, string |
Type of the Scheme Token.
Possible values: APPLEPAY, GOOGLEPAY, SAMSUNGPAY, CLICKTOPAY, OTHER, MDES, VTS. |
Container "Payment_Models_Data_StrongCustomerAuthenticationDirect"
Exemption string |
Type of Exemption
Possible values: LOW_VALUE, TRANSACTION_RISK_ANALYSIS, RECURRING. |
IssuerReference object |
Contains data that is received from the issuer in the response of a successful payment by other payment providers and will be forwarded to scheme for only this payment.
|
Container "Payment_Models_Data_StrongCustomerAuthenticationInteractive"
Exemption string |
Type of Exemption
Possible values: LOW_VALUE, TRANSACTION_RISK_ANALYSIS. |
ThreeDsChallenge string |
3DS Secure challenge options
Possible values: FORCE. |
Container "Payment_Models_Data_StrongCustomerAuthenticationReferenced"
Exemption string |
Type of Exemption
Possible values: RECURRING. |
Container "Payment_Models_Data_ThreeDsInfo"
Authenticated mandatory, boolean |
Indicates, whether the payer has successfuly authenticated him/herself or not.
|
AuthenticationType string |
Determines how the card holder was authenticated. Some 3D Secure Versions allow a Frictionless authentication.
Possible values: STRONG_CUSTOMER_AUTHENTICATION, FRICTIONLESS, ATTEMPT, EXEMPTION, NONE.Example: StrongCustomerAuthentication
|
Version string |
The 3D Secure Version.
Example: 2
|
Xid mandatory, string |
Transaction Id, given by the MPI. References the 3D Secure process.
Example: ARkvCgk5Y1t/BDFFXkUPGX9DUgs= for 3D Secure version 1 /
1ef5b3db-3b97-47df-8272-320d0bd18ab5 for 3D Secure version 2
|
Container "Payment_Models_Data_Transaction"
AcquirerName string |
Name of the acquirer
Example: AcquirerName
|
AcquirerReference string |
Reference id of the acquirer (if available)
Example: AcquirerReference
|
Amount mandatory, object |
Amount (currency, value, etc.) that has been authorized.
|
ApprovalCode string |
Approval id of the acquirer (if available)
Example: 109510
|
CaptureId string |
Unique Saferpay capture id.
Id[1..64]Available if the transaction was already captured (Status: CAPTURED). Must be stored for later reference (eg refund). Example: ECthWpbv1SI6SAIdU2p6AIC1bppA
|
Date mandatory, date |
Date / time of the authorization
Example: 2011-09-23T14:57:23.023+02.00
|
DirectDebit object |
Direct Debit information, if applicable
Example: AcquirerReference
|
Id mandatory, string |
Unique Saferpay transaction id. Used to reference the transaction in any further step.
Example: K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Invoice object |
Invoice information, if applicable
|
IssuerReference object |
Issuer reference information, if applicable
|
OrderId string |
OrderId given with request
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
SixTransactionReference mandatory, string |
Unique SIX transaction reference.
Example: 0:0:3:K5OYS9Ad6Ex4rASU1IM1b3CEU8bb
|
Status mandatory, string |
Current status of the transaction. One of 'AUTHORIZED','CANCELED', 'CAPTURED' or 'PENDING'
Possible values: AUTHORIZED, CANCELED, CAPTURED, PENDING.Example: AUTHORIZED
|
Type mandatory, string |
Type of transaction. One of 'PAYMENT', 'REFUND'
Possible values: PAYMENT, REFUND.Example: PAYMENT
|
Container "Payment_Models_Data_TransactionNotification"
PayerDccReceiptEmail string |
Email address to which a confirmation email will be sent to the payer after successful authorizations processed with DCC.
Example: payer@saferpay.com
|
Container "Payment_Models_Data_TransactionReference"
OrderId string |
Unambiguous OrderId of the referenced transaction.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
TransactionId string |
Id of the referenced transaction.
AlphaNumeric[1..64]Example: 723n4MAjMdhjSAhAKEUdA8jtl9jb
|
Container "Payment_Models_Data_TwintInfo"
CertificateExpirationDate mandatory, date |
Twint token expiry date
Example: 2019-11-08T12:29:37.000+01:00
|
Container "Payment_Models_Data_UpdateAlias"
Id mandatory, string |
The id of the alias that should be updated.. This value is case-insensitive.
Id[1..40]Example: alias35nfd9mkzfw0x57iwx
|
Lifetime integer |
Number of days this card is to be stored within Saferpay. If not filled, the default lifetime (1096 days) will be used.
Range: inclusive between 1 and 1600Example: 1000
|
Container "Payment_Models_Data_UpdateCreditCard"
ExpMonth mandatory, integer |
Month of expiration (eg 9 for September)
Range: inclusive between 1 and 12Example: 9
|
ExpYear mandatory, integer |
Year of expiration
Range: inclusive between 2000 and 9999Example: 2015
|
Container "Payment_Models_Data_UpdatePaymentMeans"
Card mandatory, object |
Card data
|
Container "Payment_Models_FraudPrevention"
Result string |
The result of the performed fraud prevention check
Possible values: APPROVED, CHALLENGED.Example: APPROVED
|
Container "Payment_Models_PaymentMethodsOptions"
Ideal object |
Optional. Options which only apply to IDEAL.
|
Klarna object |
Optional. Options which only apply to Klarna.
|
Container "Payment_Models_RefundPaymentMethodsOptions"
A2A object |
Optional. Options which only apply to account to account
|
Container "Payment_Models_RiskDetails"
BlockReason string |
Indicates the blocking reason of the transaction.
Possible values: BLACKLIST_IP, BLACKLIST_IP_ORIGIN, BLACKLIST_PAYMENT_MEANS, BLACKLIST_PAYMENT_MEANS_ORIGIN.Example: BLACKLIST_IP_ORIGIN
|
IpLocation string |
Is the location of the payer and it can be either the two letter country-code (such as CH, DE, etc.)
Example: or the special cases. The special cases are: PROXY and SATELLITE_PROVIDER. CH
|
Container "RestApi_Models_Data_Order"
Items mandatory, array of objects |
Order items
|
Container "RestApi_Models_Data_OrderItem"
CategoryName string |
Category name
Utf8[1..100]Example: knives
|
Description string |
Product description
Utf8[1..200]Example: The well known swiss army knife
|
DiscountAmount string |
Discount amount including tax
Example: 10
|
Id string |
Product id
Utf8[1..100]Example: C123192
|
ImageUrl string |
URL to an image showing the product
Max length: 2000Example: https://merchanthost/product/1/image
|
IsPreOrder boolean |
Flag, which indicates that the order item is a pre order. Per default, it is not a pre order.
Example: true
|
Name string |
Product name
Utf8[1..200]Example: red swiss army knife
|
ProductUrl string |
URL to the product page
Max length: 2000Example: https://merchanthost/product/1
|
Quantity integer |
The quantity of the order item
Example: 3
|
TaxAmount string |
Tax amount which is included in the item price
Example: 42
|
TaxRate string |
Tax rate of the item price in hundredth of a percent. e.g. value 125 means 1.25%
Range: inclusive between 0 and 99999Valid values are 0-99999 Example: 2100
|
Type string |
Order item type
Possible values: DIGITAL, PHYSICAL, SERVICE, GIFTCARD, DISCOUNT, SHIPPINGFEE, SALESTAX, SURCHARGE. |
UnitPrice string |
Price per single item in minor unit (CHF 2.00 ⇒ Value=200). Only Integer values will be accepted!
Example: 200
|
VariantId string |
Product variant id
Utf8[1..100]Example: C123192-red
|
Container "RestApi_Models_Data_PaymentWithOptions"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description mandatory, string |
A human readable description provided by the merchant that will be displayed in Payment Page.
Utf8[1..100]Example: Description of payment
|
Options object |
Specific payment options
|
OrderId mandatory, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
Container "RestApi_Models_Data_PaymentWithoutOptions"
Amount mandatory, object |
Amount data (currency, value, etc.)
|
Description mandatory, string |
A human readable description provided by the merchant that will be displayed in Payment Page.
Utf8[1..100]Example: Description of payment
|
OrderId mandatory, string |
Unambiguous order identifier defined by the merchant / shop. This identifier might be used as reference later on.
Id[1..80]Example: c52ad18472354511ab2c33b59e796901
|
Container "RestApi_Models_Data_RegisterAlias"
IdGenerator mandatory, string |
Id generator to be used by Saferpay.
Possible values: RANDOM.Example: RANDOM
|
Container "RestApi_Models_Feature"
DisplayName mandatory, string |
Display name of the feature
Example: Saferpay Example
|
Id mandatory, string |
Id of the feature
Example: SAFERPAY_EXAMPLE
|
Container "RestApi_Models_LicensePackage"
DisplayName mandatory, string |
Display name of the package
Example: Saferpay Example
|
Id mandatory, string |
Id of the package
Example: SAFERPAY_EXAMPLE
|
Container "RestApi_Models_PaymentMethodInfo"
Currencies array of strings |
Array of strings representing all the supported currencies for the payment method
|
LogoUrl string |
The logo of the payment method as url
|
PaymentMethod string |
Name of the payment method
|
Container "RestApi_Models_TerminalResult"
Description string |
Description of the terminal
|
PaymentMethods array of objects |
Array of payment methods that are available for the terminal
|
TerminalId string |
The Id of the terminal
|
Type string |
The type of the terminal
Possible values: ECOM, SPG, MPO. |
Wallets array of objects |
Array of wallets that are available for the terminal
|
Container "RestApi_Models_WalletInfo"
LogoUrl string |
The logo of the wallet as url
|
WalletName string |
Name of the wallet
|
Changelog
Table of Contents
- Version 1.41
- Version 1.40
- Version 1.39
- Version 1.38
- Version 1.37
- Version 1.36
- Version 1.35
- Version 1.34
- Version 1.33
- Version 1.32
- Version 1.31
- Version 1.30
- Version 1.29
- Version 1.28
- Version 1.27
- Version 1.26
- Version 1.25
- Version 1.24
- Version 1.23
- Version 1.22
- Version 1.21
- Version 1.20
- Version 1.19
- Version 1.18
- Version 1.17
- Version 1.16
- Version 1.15
- Version 1.14
- Version 1.13
- Version 1.12
- Version 1.11
- Version 1.10
- Version 1.9
- Version 1.8
- Version 1.7
- Version 1.6
- Version 1.5
- Version 1.4
Version 1.41 (released 2024-07-16)
- available on Sandbox: 2024-07-02
- introduced version 1.41
- added value
1.41
for SpecVersion - added additional info about the used ISO-standard and applied restrictions to field
LanguageCode
of the Payer container and SecureCardData/AliasInsert requests - added new subcontainer ForeignRetailer to container Marketplace. This affects the following requests:
Version 1.40 (released 2024-05-21)
- available on Sandbox: 2024-05-07
- introduced version 1.40
- added value
1.40
for SpecVersion - added Scheme Token type
MDES
andVTS
(only available for Worldline Acquiring) - added
WECHATPAY
as valid value for the fieldPaymentMethods
- added
SchemeToken
toPaymentMeans
container - added
POSTFINANCEPAY
as valid value forType
in Alias/Insert - removed
PAYPAL
as option in Transaction/RedirectPayment (feature is deprecated) - removed
POSTFINANCE
andPOSTCARD
as valid values forPaymentMethods
in PaymentPage/Initialize requests - removed
POSTFINANCE
as valid value forType
in Alias/Insert - removed
BANCONTACT
as valid value forPaymentMethods
in Alias/Insert
Version 1.39 (released 2024-03-19)
- available on Sandbox: 2024-03-01
- introduced version 1.39
- added value
1.39
for SpecVersion - removed
Styling
container from PaymentPage/Initialize in all API versions - PaymentPage/Assert can now be called up to 120 hours for pending transactions (instead of 96 hours before)
- removed
Transaction/QueryPaymentMeans
andTransaction/AdjustAmount
methods from Saferpay Payment API - added
MandatoryFields
field to BillingAddressForm container in SecurePayGate Create SingleUsePaymentLink - option
MANUAL_REVIEW
in fieldResult
of FraudPrevention container is now calledCHALLENGED
. This affects following requests:
Version 1.38 (released 2024-01-23)
- available on Sandbox: 2024-01-04
- introduced version 1.38
- added value
1.38
for SpecVersion - added 48 bits support to
ColorDepth
- removed Alipay from
PaymentMethodOptions
container PaymentPage/Initialize - added
PaymentMethodsOptions
to Transaction/Refund for A2A AccountHolderName - added
WITH_SUCCESSFUL_THREE_DS_CHALLENGE
value toCondition
in SecurePayGate Create SingleUsePaymentLink requests - Token expiration increased for PaymentPage/Assert and pending transactions
- improve case validation
- common error message improvements
Version 1.37 (released 2023-11-14)
- available on Sandbox: 2023-10-27
- introduced version 1.37
- added value
1.37
for SpecVersion - added
POSTFINANCEPAY
as valid value for fieldPaymentMethods
in PaymentPage/Initialize requests - extended selection of possible characters for
PayerId
, affecting the following requests and responses:- PaymentPage/Initialize
- PaymentPage/Assert
- Transaction/Initialize
- Transaction/Authorize
- Transaction/QueryPaymentMeans
- Transaction/AuthorizeDirect
- Transaction/AuthorizeReferenced
- Transaction/RedirectPayment
- Transaction/AssertRedirectPayment
- Transaction/Inquire
- Transaction/AlternativePayment
- Transaction/QueryAlternativePayment
- added
TokenType
field toSchemeToken
, affecting the following requests and responses:
Version 1.36 (released 2023-09-12)
- available on Sandbox: 2023-08-29
- introduced version 1.36
- added value
1.36
for SpecVersion - removed
CheckResult
from OmniChannel/InsertAlias response - removed
UNIONPAY
as valid value from fieldPaymentMethods
in Transaction/Initialize requests - removed
LegalForm
from theBillingAddress
,DeliveryAddress
andRecipient
container, affecting the following requests and responses:- PaymentPage/Initialize
- PaymentPage/Assert
- Transaction/Initialize
- Transaction/Authorize
- Transaction/QueryPaymentMeans
- Transaction/AuthorizeDirect
- Transaction/AuthorizeReferenced
- Transaction/RefundDirect
- Transaction/RedirectPayment
- Transaction/AssertRedirectPayment
- Transaction/Inquire
- Transaction/AlternativePayment
- Transaction/QueryAlternativePayment
- OmniChannel/AcquireTransaction
- SecurePayGate Create SingleUsePaymentLink
- renamed the following values of the
Condition
fieldWITH_LIABILITY_SHIFT
is nowTHREE_DS_AUTHENTICATION_SUCCESSFUL_OR_ATTEMPTED
IF_ALLOWED_BY_SCHEME
is nowNONE
- added
Condition
field to SecurePayGate Create SingleUsePaymentLink requests
Version 1.35 (released 2023-07-18)
- available on Sandbox: 2023-07-04
- introduced version 1.35
- added value
1.35
for SpecVersion - added CARD as value from field PaymentMethod in PaymentPage/Initialize requests
- added CustomerLicense method to Saferpay Management API
- removed
CustomerLicenseConfiguration
method from Saferpay Management API - added endpoint SecurePayGate
Delete
SingleUsePaymentLink to Saferpay Management API - added container
payer
to Transaction/Authorize and Transaction/AuthorizeDirect - added container
notification
to Transaction/Initialize and Transaction/AuthorizeReferenced - added property
PayerDccReceiptEmail
to PaymentPage/Initialize, Transaction/Initialize and Transaction/AuthorizeReferenced - per default, in PaymentPage/Initialize and Transaction/Initialize the field
HolderName
is not shown - SecurePayGate Create SingleUsePaymentLink, argument
ExpirationDate
is now in the formatyyyy-MM-ddTHH:mm:ssK
and has to be at least 1h in the future and within 180 days - the
CountrySubdivisionCode
in the address containers now also accepts complete country codes (e.g.CH-ZH
) beside the already accepted subdivision codes
Version 1.34 (released 2023-05-16)
- available on Sandbox: 2023-05-03
- introduced version 1.34
- added value
1.34
for SpecVersion - added parameter
DeviceFingerprintTransactionId
to container RiskFactors in PaymentPage/Initialize, Transaction/Initialize, Transaction/AuthorizeDirect and Transaction/AlternativePayment requests - removed
MASTERPASS
as valid value from fieldWallets
in PaymentPage/Initialize requests - removed
UNIONPAY
as valid value from fieldPaymentMethods
in SecureCardData/AliasInsert requests - added additional info about expected values to field
LanguageCode
of the Payer container and SecureCardData/AliasInsert requests - updated link to license information
Version 1.33 (released 2023-03-21)
- available on Sandbox: 2023-03-07
- introduced version 1.33
- added value
1.33
for SpecVersion - added
NotifyUrl
to the SecureCardData/AliasInsert request - added the value
FraudPrevention
to the Transaction/Inquire response - added
ACCOUNTTOACCOUNT
as a possible payment method in PaymentPage/Initialize and PaymentPage/Assert - a new method was added which allows the merchant to fetch data about a previously created SingeUsePaymentLink:
/rest/customers/{customerId}/terminals/{terminalId}/spg-offers/{offerId}
- the error behavior
ABORT
was renamed toDO_NOT_RETRY
- the new error message
UPDATE_CARD_INFORMATION
was added DeviceFingerprint
was removed from all versions- the
Twint
object was removed from the response of:
Version 1.32 (released 2023-01-17)
- available on Sandbox: 2022-12-29
- introduced version 1.32
- added value
1.32
for SpecVersion - this method is now obsolete:
/api/rest/customers/{customerid}/terminals/{terminalid}/payment-methods
and replaced with this method:/api/rest/customers/{customerid}/terminals/{terminalid}
- in addition, there is a new method that allows the merchant to query which terminals he has:
/api/rest/customers/{customerid}/terminals
- added values
PAYCONIQ
for Brand.PaymentMethod and PaymentMethods in PaymentPage/Initialize - container ReturnUrls (parameters Success and Fail) is replaced by container ReturnUrl (parameter Url)
Version 1.31 (released 2022-11-15)
- available on Sandbox: 2022-11-01
- introduced version 1.31
- added value
1.31
for SpecVersion - added new function to Saferpay Management API for querying the license configuration of a customer
Version 1.30 (released 2022-09-20)
- available on Sandbox: 2022-09-06
- introduced version 1.30
- added value
1.30
for SpecVersion - added ProductUrl and ImageUrl to
Order Items
container in PaymentPage/Initialize - added OrderId in the response to PaymentPage/Assert and Transaction/Authorize
Version 1.29 (released 2022-07-19)
- available on Sandbox: 2022-07-19
- introduced version 1.29
- added value
1.29
for SpecVersion - in addition to Transaction/AuthorizeDirect, partial approval is now supported by Transaction/Initialize. Using this option in Transaction/Initialize prevents DCC.
Version 1.28 (released 2022-05-17)
- available on Sandbox: 2022-05-04
- introduced version 1.28
- added value
1.28
for SpecVersion - added parameter VatNumber to
BillingAddress
container in PaymentPage/Initialize, Transaction/Initialize, Transaction/AuthorizeDirect, Transaction/AlternativePayment, Secure PayGate API SecurePayGateOffer CreateOffer requests and PaymentPage/Assert, Transaction/Authorize, Transaction/QueryPaymentMeans, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/Inquire and Transaction/QueryAlternativePayment responses
Version 1.27 (released 2022-03-15)
- available on Sandbox: 2022-03-01
- introduced version 1.27
- added value
1.27
for SpecVersion - container Liability replaces ThreeDs in Transaction/AuthorizeDirect responses
- added parameters Markup and ExchangeRate to container Dcc in PaymentPage/Assert, Transaction/Authorize, Transaction/AuthorizeReferenced, Transaction/Refund and Transaction/Inquire responses
- added parameter Email to container PayPal in PaymentPage/Assert and Transaction/Inquire responses
- added parameter DeviceFingerprint to container RiskFactors in PaymentPage/Initialize, Transaction/Initialize, Transaction/AuthorizeDirect and Transaction/AlternativePayment requests
- added containers ApplePay and GooglePay to Alias/InsertDirect requests
- added container ApplePay to Transaction/Initialize requests
- added values
GOOGLEPAY
andAPPLEPAY
toWallet
in Transaction/AuthorizeDirect responses - added value
APPLEPAY
toWallet
in Transaction/Authorize responses
Version 1.26 (released 2022-01-18)
- available on Sandbox: 2021-12-30
- introduced version 1.26
- added value
1.26
for SpecVersion - added container GooglePay to Transaction/Initialize requests
- added parameter AddressSource to container BillingAddressForm in PaymentPage/Initialize and Secure PayGate API SecurePayGateOffer CreateOffer
- added parameter AddressSource to container DeliveryAddressForm in PaymentPage/Initialize
- removed parameter Display from containers BillingAddressForm and DeliveryAddressForm in PaymentPage/Initialize and Secure PayGate API SecurePayGateOffer CreateOffer as it is replaced by AddressSource
Version 1.25 (released 2021-11-16)
- available on Sandbox: 2021-11-02
- introduced version 1.25
- added value
1.25
for SpecVersion - added value
GOOGLEPAY
for Wallets in PaymentPage/Initialize request to enable the preselection of Apple Pay - added container PayPal to PaymentPage/Assert response
- added parameter RestrictRefundAmountToCapturedAmount to container Refund in Transaction/Refund request
- added parameter LogoUrl to containers PaymentMethods and Wallets in Management API TerminalInfo PaymentMethods response
Version 1.24 (released 2021-09-14)
- available on Sandbox: 2021-08-31
- introduced version 1.24
- added value
1.24
for SpecVersion - replaced parameter
NotifyUrl
in the Notification container with the two separate parametersSuccessNotifyUrl
andFailNotifyUrl
in PaymentPage/Initialize and Transaction/RedirectPayment requests - added container Klarna to PaymentPage/Initialize request
- added container OriginalCreditTransfer to Transaction/RefundDirect request
- added container ApplePay to Transaction/Initialize, Transaction/AuthorizeDirect and Alias/InsertDirect requests
Version 1.23 (released 2021-07-13)
- available on Sandbox: 2021-07-13
- introduced version 1.23
- added value
1.23
for SpecVersion - added container RedirectNotifyUrls to Transaction/Initialize request
- added container SchemeToken to Transaction/Initialize, Transaction/AuthorizeDirect, Transaction/RefundDirect and Alias/InsertDirect requests
- added container IssuerReference to Alias/InsertDirect request
- removed parameter VerificationValue from PaymentPage/Assert, Transaction/Authorize, Transaction/Inquire, Transaction/QueryAlternativePayment, Alias/AssertInsert, Alias/InsertDirect and OmniChannel/InsertAlias responses
Version 1.22 (released 2021-05-18)
- available on Sandbox: 2021-05-04
- introduced version 1.22
- added value
1.22
for SpecVersion - changed values of parameter LiableEntity to ALL_CAPS instead of PascalCase in PaymentPage/Assert, Transaction/Authorize, Transaction/Inquire and Transaction/QueryAlternativePayment responses
- added parameter InPsd2Scope to container Liability in PaymentPage/Assert, Transaction/Authorize, Transaction/Inquire and Transaction/QueryAlternativePayment responses
- added parameters Version and AuthenticationType to container ThreeDs in PaymentPage/Assert, Transaction/Authorize, Transaction/Inquire and Transaction/QueryAlternativePayment responses
Version 1.21 (released 2021-03-23)
- available on Sandbox: 2021-03-09
- introduced version 1.21
- added value
1.21
for SpecVersion - added new function to Saferpay Management API for automated creation of Saferpay Fields Access Tokens
- added parameter Initiator to Transaction/AuthorizeDirect request
- added container IssuerReference to Transaction/AuthorizeDirect request and PaymentPage/Assert, Transaction/Authorize, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/Refund, Transaction/RefundDirect, Transaction/AssertRedirectPayment, Transaction/Inquire, Transaction/QueryAlternativePayment and OmniChannel/AcquireTransaction responses
- added container Risk to the Error Message
- added parameter PayerMessage to the Error Message
Version 1.20 (released 2020-11-10)
- available on Sandbox: 2020-10-27
- introduced version 1.20
- added value
1.20
for SpecVersion - added containers Order and RiskFactors to Transaction/AuthorizeDirect and Transaction/AlternativePayment requests
- added AllowPartialAuthorization flag in Options container under Payments in Transaction/AuthorizeDirect
- added container FraudPrevention to Transaction/Authorize, Transaction/AuthorizeDirect and Transaction/QueryAlternativePayment responses
Version 1.19 (released 2020-09-15)
- available on Sandbox: 2020-09-01
- introduced version 1.19
- added value
1.19
for SpecVersion - added container PayerProfile to container RiskFactors in PaymentPage/Initialize and Transaction/Initialize requests
- added containers Order and RiskFactors to Transaction/AlternativePayment request
- added container FraudPrevention in Transaction/Authorize, PaymentPage/Assert and Transaction/AlternativePayment responses
- removed parameters AccountCreationDate and PasswordLastChangeDate from container RiskFactors in PaymentPage/Initialize and Transaction/Initialize requests (moved to container PayerProfile)
- added parameter Description to container Payment in Saferpay Secure PayGate API CreateOffer request
Version 1.18 (released 2020-07-14)
- available on Sandbox: 2020-07-01
- introduced version 1.18
- added value
1.18
for SpecVersion - added parameter Id to container Payer in PaymentPage/Initialize, Transaction/Initialize, Transaction/AuthorizeDirect, Transaction/RedirectPayment, Transaction/AlternativePayment requests and PaymentPage/Assert, Transaction/Authorize, Transaction/QueryPaymentMeans, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/AssertRedirectPayment, Transaction/Inquire, Transaction/QueryAlternativePayment responses
- added container Order in PaymentPage/Initialize and Transaction/Initialize requests
- added container RiskFactors in PaymentPage/Initialize and Transaction/Initialize requests
- added container RegisterAlias in Secure PayGate API SecurePayGateOffer CreateOffer
Version 1.17 (released 2020-05-12)
- available on Sandbox: 2020-04-28
- introduced version 1.17
- added value
1.17
for SpecVersion - added parameter VerificationCode to container CardForm in Transaction/Initialize request
- added container SaferpayFields in Alias/Insert and Alias/InsertDirect requests
- removed parameter RedirectUrl in Alias/Insert response
- added parameter RedirectRequired in Alias/Insert response
- added container Redirect in Alias/Insert response
- marked parameter CssUrl as deprecated in PaymentPage/Initialize
Version 1.16 (released 2020-03-17)
- available on Sandbox: 2020-03-03
- introduced version 1.16
- added value
1.16
for SpecVersion - added value
ONLINE_STRONG
for Check.Type in Alias/Insert request - added value
OK_AUTHENTICATED
for CheckResult.Result in Alias/AssertInsert response - added container Authentication in PaymentPage/Initialize, Transaction/Initialize, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced requests
- added container Authentication to container CheckResult in Alias/AssertInsert, Alias/InsertDirect and OmniChannel/InsertAlias responses
- added container AuthenticationResult in PaymentPage/Assert, Transaction/Authorize and Transaction/AuthorizeDirect responses
- added container MastercardIssuerInstallments in PaymentPage/Assert, Transaction/Authorize and Transaction/AuthorizeDirect responses
- added container MastercardIssuerInstallments in Transaction/Capture request
Version 1.15 (released 2020-01-21)
- available on Sandbox: 2020-01-07
- introduced version 1.15
- added value
1.15
for SpecVersion - added container Ideal to PaymentPage/Initialize request to enable the preselection of an iDEAL issuer
- added value
APPLEPAY
for Wallets in PaymentPage/Initialize request to enable the preselection of Apple Pay
Version 1.14 (released 2019-11-19)
- available on Sandbox: 2019-11-05
- introduced version 1.14
- added value
1.14
for SpecVersion - added new Saferpay Secure PayGate API for automated creation of Saferpay Secure PayGate offers
- added container SaferpayFields to container PaymentMeans in Transaction/Initialize, Transaction/AuthorizeDirect and Transaction/RefundDirect requests
Version 1.13 (released 2019-09-17)
- available on Sandbox: 2019-09-10
- introduced version 1.13
- added value
1.13
for SpecVersion - added container PaymentMethodsOptions to Transaction/AlternativePayment to allow the setting of payment method specific options. Currently used for Bancontact specific settings
- added new country code value XK (Kosovo) to field CountryCode
- added new value DIVERSE in field Gender
- added new error code PAYMENTMEANS_NOT_SUPPORTED with corresponding error message "Unsupported means of payment (e.g. non SEPA IBAN)"
Version 1.12 (released 2019-07-16)
- available on Sandbox: 2019-07-02
- introduced version 1.12
- added value
1.12
for SpecVersion - added method Alias/Update for updating the details of an alias
- added methods Transaction/AlternativePayment and Transaction/QueryAlternativePayment which enable the implementation of customized checkout processes (i.e. in mobile shopping apps) - at the moment only available for Bancontact
- replaced MerchantEmail with MerchantEmails that can be filled with up to 10 email addresses to which the payment notification is sent
Version 1.11 (released 2019-05-21)
- available on Sandbox: 2019-05-07
- introduced version 1.11
- added value
1.11
for SpecVersion - added method Transaction/Inquire for inquiring the details of previous transaction
- added container PaymentMethodsOptions to PaymentPage/Initialize to allow for setting payment method specific options
- added Condition to PaymentPage/Initialize request to control the minimum authentication level
- added HolderSegment to Alias/AssertInsert and Alias/InsertDirect responses to indicate the segment (e.g. Corporate) of the card holder
- added CaptureId to PaymentPage/Assert, Transaction/Authorize, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/Refund, Transaction/RefundDirect and OmniChannel/AcquireTransaction responses to identify a (partial) capture for refunding
- added value
IF_ALLOWED_BY_SCHEME
for fieldCondition
in Transaction/Authorize request - added value
ALIPAY
for Brand.PaymentMethod, PaymentMethods and Wallet.PaymentMethods - removed
VerificationValue
fromThreeDs
container
Version 1.10 (released 2018-11-13)
- available on Sandbox: 2018-08-14
- introduced version 1.10
- added value
1.10
for SpecVersion - added method Transaction/MultipartCapture for capturing multiple parts of a transaction also supporting enhanced clearing for marketplaces
- added method Transaction/MultipartFinalize to finalize a transaction that is still open for capturing additional parts
- added container MarketPlace to Transaction/Capture request to support enhanced clearing for marketplaces
- removed container Partial from Transaction/Capture request - see method Transaction/MultipartCapture for details
- replaced TransactionId and OrderId with CaptureId in Transaction/Capture response to uniquely identify captures
- replaced container TransactionReference with CaptureReference in Transaction/Refund and Transaction/AssertCapture request to uniquely identify captures
- added value
TWINT
for fieldType
in Alias/Insert requests (only available in the Sandbox environment until further notice) - added subcontainer
Twint
to containerPaymentMeans
Version 1.9 (released 2018-05-15)
- available on Sandbox: 2018-04-26
- introduced version 1.9
- added value
1.9
for SpecVersion - replaced container ThreeDs from previous versions with Liability in PaymentPage/Assert and Transaction/Initialize responses
Version 1.8 (released 2017-11-14)
- available on Sandbox: 2017-11-02
- introduced version 1.8
- added value
1.8
for SpecVersion - added SixTransactionReference to PaymentPage/Assert, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/RefundDirect and Transaction/Refund responses for Omni Channel use cases
- added method OmniChannel/AcquireTransaction for retrieving a previously authorized transaction from another channel
- added method OmniChannel/InsertAlias for retrieving an alias for a card used in a previously authorized transaction from another channel
- added container CardForm for Alias/Insert and Transaction/Initialize requests for adjusting the card form's mandatory fields
Version 1.7 (released 2017-08-22)
- available on Sandbox: 2017-08-03
- introduced version 1.7
- added value
1.7
for SpecVersion - added value
TWINT
for Brand.PaymentMethod - added ApprovalCode to PaymentPage/Assert, Transaction/AuthorizeDirect, Transaction/AuthorizeReferenced, Transaction/RefundDirect and Transaction/Refund responses
- added PaymentMethods to Transaction/Initialize and Alias/Insert requests
- increased number of concurrent Basic Authentication credentials to 10
- improved description for pending statuses
Version 1.6 (released 2017-04-04)
- available on Sandbox: 2017-03-14
- introduced version 1.6
- added value
1.6
for SpecVersion - added container Check for Alias/InsertDirect request
- added container CheckResult for Alias/AssertInsert and Alias/InsertDirect responses
Version 1.5 (released 2017-02-07)
- introduced version 1.5
- added value
1.5
for SpecVersion - added method Transaction/AssertCapture for checking the status of a pending capture (currently needed for paydirekt)
- added method Transaction/AssertRefund for checking the status of a pending refund (currently needed for paydirekt)
- added container PendingNotification for Transaction/Capture requests for notification settings on pending captures (currently needed for paydirekt)
- added container PendingNotification for Transaction/Refund requests for notification settings on pending captures (currently needed for paydirekt)
- added Status in Transaction/Capture responses to indicate the status of a capture
- changed Date in Transaction/Capture responses to optional, e.g. for pending captures
- changed PaymentPage/Initialize request to allow BillingAddressForm.Display and DeliveryAddressForm.Display both set to
true
at the same time - added ContentSecurityEnabled to Styling container to control Content Security Policy
- corrected type documentation for CountrySubdivisionCode to "AlphaNumeric" in the address containers
Version 1.4 (released 2016-10-15)
- new version 1.4
- added value
1.4
for SpecVersion - added option SuppressDcc for Transaction/AuthorizeReferenced
- added values
BANCONTACT
andPAYDIREKT
for Brand.PaymentMethod and PaymentMethods in PaymentPage/Initialize - added validation for element Payment.MandateId
- added option ConfigSet for PaymentPage/Initialize
- added value
- added a note that partial captures are currently only supported for PayPal
- added a note that values for AliasID are case-insensitive
- corrected example for Transaction/AuthorizeDirect
- corrected example for Transaction/AuthorizeReferenced
- corrected example for Transaction/Authorize
- corrected example for Transaction/Initialize
- improved description for TransactionReference
- corrected example for BillpayCapture.DelayInDays
- improved description for ReturnUrls